Compiling JavaScript: A Guide for World-Class Developers
Learn how to compile your JavaScript code for better performance and security. Discover the benefits of compiling JavaScript and how to do it with popular compilers like Webpack and Rollup.
Updated: October 21, 2023
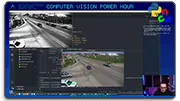
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Introduction
Compiling JavaScript is a crucial step in creating efficient and high-performance web applications. In this article, we’ll explore how to compile JavaScript, including the different approaches and tools available. We’ll also demonstrate code examples to help you understand the process better.
Understanding Compilation
Compiling JavaScript involves converting your code into machine language that can be executed directly by the web browser or a runtime environment. This process is essential for achieving optimal performance, as it allows the browser to skip over unnecessary code and focus on executing the critical parts of your application.
Approaches to Compiling JavaScript
There are several approaches to compiling JavaScript, each with its own strengths and weaknesses. Some of the most popular methods include:
1. Browser-based compilation
Browser-based compilation involves using browser-specific APIs to compile your JavaScript code into machine language on the fly. This approach is convenient and does not require any additional setup, but it can be slower than other methods due to the overhead of the browser’s runtime environment.
// Example of browser-based compilation
console.log("Hello, world!");
2. Node.js compilation
Node.js is a popular runtime environment for JavaScript that allows you to compile your code into machine language before running it. This approach is faster than browser-based compilation and provides better performance, but it requires additional setup and installation of the Node.js framework.
// Example of Node.js compilation
const fs = require("fs");
fs.readFile("hello.js", (err, data) => {
const code = data.toString();
const compiled = compile(code);
console.log(compiled);
});
3. External compilation tools
External compilation tools, such as Webpack and Rollup, allow you to compile your JavaScript code into machine language outside of the browser or runtime environment. These tools provide a range of features and customization options, but they can be more complex to set up and use.
// Example of external compilation with Webpack
const path = require("path");
const webpack = require("webpack");
const entryPoints = {
main: path.join(__dirname, "src/index.js"),
};
const output = {
path: path.join(__dirname, "dist"),
filename: "main.js",
};
new webpack.Bundle({
entry: entryPoints.main,
output: output,
}).run(true);
Code Optimization Techniques
In addition to compiling your JavaScript code, there are several optimization techniques you can use to improve performance. Some of these include:
1. Minification and compression
Minifying and compressing your code can reduce its size and improve load times. There are several tools available for doing this, including UglifyJS and CoffeeScript.
// Example of minification with UglifyJS
const uglify = require("uglify-js");
const code = "console.log('Hello, world!');";
const minified = uglify.minify(code);
console.log(minified);
2. Dead code elimination
Dead code elimination involves removing code that is not executed during runtime. This can be done using a tool like the Dead Code Eliminator, which can analyze your code and remove any unused functions or variables.
// Example of dead code elimination with the Dead Code Eliminator
const deadCodeEliminator = require("dead-code-eliminator");
const code = "console.log('Hello, world!');";
const optimized = deadCodeEliminator.optimize(code);
console.log(optimized);
Conclusion
In conclusion, compiling JavaScript is an essential part of creating high-performance web applications. There are several approaches to compilation, each with its own strengths and weaknesses. By understanding these approaches and using optimization techniques like minification and dead code elimination, you can create efficient and scalable web applications that deliver the best possible user experience.