Building a Website with JavaScript
Learn how to build a website using JavaScript, including how to create a basic layout, add interactivity with events and animations, and style your site with CSS. Easy-to-follow tutorials and examples for beginners and experienced developers. (196 characters)
Updated: October 20, 2023
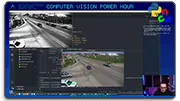
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript is a powerful programming language that is widely used for building dynamic and interactive web applications. In this article, we will explore the basics of building a website using JavaScript, including setting up the basic structure of the site, adding interactivity with JavaScript, and incorporating additional features such as animations and effects.
Setting Up the Basic Structure of the Site
Before we dive into the JavaScript code, let’s first set up the basic structure of our website. We will use HTML to define the content and structure of our site, and CSS to style it. Here is an example of the basic structure of a simple website:
<!DOCTYPE html>
<html>
<head>
<title>My Website</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
<main>
<section id="home">
<h2>Home</h2>
<p>This is the home section of my website.</p>
</section>
<section id="about">
<h2>About</h2>
<p>This is the about section of my website.</p>
</section>
</main>
</body>
</html>
Adding Interactivity with JavaScript
Now that we have our basic structure set up, let’s add some interactivity using JavaScript. We will start by adding a simple click event listener to our website’s navigation menu. Here is an example of how to do this:
<script>
// Get all the navigation links
var navLinks = document.querySelectorAll(".nav-link");
// Add a click event listener to each link
navLinks.forEach(function(link) {
link.addEventListener("click", function() {
// Switch the current section to the one that was clicked
var currentSection = document.querySelector(this.getAttribute("data-section"));
document.body.appendChild(currentSection);
});
});
</script>
In this code, we first get all the navigation links using document.querySelectorAll()
and store them in an array called navLinks
. We then add a click event listener to each link using link.addEventListener()
. When a link is clicked, the function passed as the second argument is executed. In this function, we use this
to refer to the current element (the link that was clicked), and we use getAttribute()
to retrieve the “data-section” attribute of the link. This attribute contains the ID of the section that should be displayed when the link is clicked. We then use document.body.appendChild()
to add the selected section to the body of the document.
Incorporating Additional Features
Now that we have our basic structure and interactivity set up, let’s incorporate some additional features such as animations and effects. Here are a few examples:
<script>
// Fade in the navigation menu on load
var navMenu = document.querySelector(".nav-menu");
navMenu.style.opacity = "0";
setTimeout(function() {
navMenu.style.opacity = "1";
}, 500);
// Add a fade out effect to the sections when they are not currently being displayed
var sections = document.querySelectorAll(".section");
sections.forEach(function(section) {
section.style. opacity = "1";
section.addEventListener("click", function() {
section.style.opacity = "0";
setTimeout(function() {
section.style.opacity = "1";
}, 500);
});
});
</script>
In this code, we first fade in the navigation menu on load using setTimeout()
. We then add a fade out effect to each section when it is not currently being displayed. When a section is clicked, we use section.style.opacity = "0"
to fade it out, and we use setTimeout()
to fade it back in after 500 milliseconds.
Conclusion
In this article, we have covered the basics of building a website using JavaScript. We have set up the basic structure of our site using HTML and CSS, and we have added interactivity using JavaScript. We have also incorporated additional features such as animations and effects to enhance the user experience. With these skills, you can build your own dynamic and interactive web applications using JavaScript.