Mastering the Art of JavaScript Development: A Comprehensive Guide to Building Your Skills and Landing Your Dream Job
Learn the skills you need to become a successful JavaScript developer, including HTML/CSS, JavaScript fundamentals, and popular frameworks like React and Angular. Get started on your journey today!
Updated: October 19, 2023
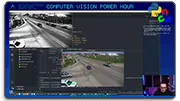
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript is one of the most popular programming languages in the world, and it’s used by millions of websites and applications. If you want to become a JavaScript developer, here are some steps you can follow:
- Learn the Basics of JavaScript
Before diving into advanced topics, it’s essential to learn the basics of JavaScript. Here are some fundamental concepts you should understand:
Variables and Data Types
In JavaScript, variables are used to store values. You can declare a variable using the var
keyword followed by the name of the variable and its data type. For example:
var myName = "John Doe";
Here, myName
is the name of the variable, and John Doe
is the value assigned to it.
Conditional Statements
Conditional statements are used to control the flow of your code based on certain conditions. Here’s an example of an if statement in JavaScript:
if (x > 5) {
console.log("x is greater than 5");
}
Here, x
is a variable that needs to be greater than 5 for the code inside the if statement to execute.
Loops
Loops are used to repeat a block of code multiple times. Here’s an example of a for loop in JavaScript:
for (var i = 0; i < 10; i++) {
console.log(i);
}
Here, the loop will iterate 10 times, and each iteration will print the current value of i
to the console.
- Learn Advanced JavaScript Concepts
Once you have a solid grasp of the basics, it’s time to move on to more advanced concepts. Here are some topics you should explore:
Functions
Functions are reusable blocks of code that can take arguments and return values. Here’s an example of a simple function in JavaScript:
function greet(name) {
console.log("Hello, " + name);
}
Here, the function greet
takes a name
argument and prints a greeting message to the console.
Object-Oriented Programming
JavaScript supports object-oriented programming (OOP) concepts like classes and inheritance. Here’s an example of a simple class in JavaScript:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log("Hello, my name is " + this.name);
}
}
Here, we define a Person
class that takes two arguments in its constructor: name
and age
. The class has a sayHello
method that prints a greeting message to the console.
Event Listeners and DOM Manipulation
JavaScript is commonly used for client-side scripting, which involves manipulating the Document Object Model (DOM) of web pages. Here’s an example of adding an event listener to a button:
const button = document.getElementById("myButton");
button.addEventListener("click", function() {
console.log("Button clicked!");
});
Here, we first get a reference to the myButton
element using document.getElementById
. We then add an event listener to the button’s click
event, which will execute the code inside the function whenever the button is clicked.
- Practice and Build Projects
The best way to learn JavaScript is by practicing and building projects. Start by building small projects like calculators, clocks, or games. As you progress, move on to more complex projects that involve more advanced concepts.
- Join Online Communities and Participate in Code Challenges
Joining online communities and participating in code challenges can help you stay motivated and learn from other developers. Some popular online communities for JavaScript developers include:
- Learn Advanced Topics and Frameworks
Once you have a solid grasp of the basics and advanced concepts, it’s time to explore more advanced topics like React, Angular, or Vue.js. These frameworks can help you build complex web applications more efficiently.
- Keep Learning and Stay Up-to-Date
Finally, it’s essential to keep learning and stay up-to-date with the latest developments in the JavaScript ecosystem. New libraries and frameworks are being developed all the time, so it’s important to stay informed and adapt your skills accordingly.
Conclusion
Becoming a JavaScript developer can be a challenging but rewarding journey. With dedication and practice, you can master the basics and advanced concepts of JavaScript and build exciting web applications that can change people’s lives. Good luck!