From Zero to Hero: How to Master JavaScript in Record Time!
Learn JavaScript quickly and effectively with our expert-led guide. Discover the best resources, tips, and techniques to master the language in no time.
Updated: October 20, 2023
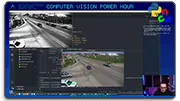
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript is a powerful and versatile programming language that is widely used in web development. If you’re interested in learning JavaScript, you might be wondering how fast you can become proficient in it. In this article, we’ll explore some tips and tricks for learning JavaScript quickly and effectively.
Tip 1: Start with the Basics
Before diving into advanced topics, it’s essential to understand the fundamentals of JavaScript. This includes understanding variables, data types, conditional statements, loops, functions, and objects. These concepts are the building blocks of JavaScript, and they will provide a solid foundation for learning more complex topics.
Here’s an example of how you can learn the basics of JavaScript:
// Variables
let name = 'John Doe';
let age = 30;
// Conditional statements
if (age >= 18) {
console.log('You are eligible to vote');
} else {
console.log('You are not eligible to vote');
}
// Loops
for (let i = 0; i < 5; i++) {
console.log(i);
}
// Functions
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet('John');
// Objects
const person = {
name: 'John Doe',
age: 30,
occupation: 'Developer'
};
console.log(person.name); // Output: John Doe
Tip 2: Practice with Real-World Projects
Once you have a solid understanding of the basics, it’s time to start practicing with real-world projects. This will help you apply your knowledge and gain practical experience. You can find many online resources that provide project ideas and tutorials, such as CodePen, FreeCodeCamp, or Udemy courses.
Here’s an example of a real-world project that you can practice:
// Create a to-do list app
// Define a function to add a new task
function addTask(title) {
const task = document.createElement('li');
task.textContent = title;
document.getElementById('todoList').appendChild(task);
}
// Add a button to the page that triggers the function
const addButton = document.createElement('button');
addButton.textContent = 'Add Task';
addButton.addEventListener('click', () => {
const title = document.getElementById('titleInput').value;
addTask(title);
});
document.getElementById('todoList').appendChild(addButton);
Tip 3: Learn by Doing
One of the most effective ways to learn JavaScript is by doing, not just reading or watching tutorials. Start by building small projects, and gradually increase the complexity as you become more comfortable with the language. This will help you apply your knowledge in real-world scenarios and gain practical experience.
Here’s an example of a simple project that you can start with:
// Create a calculator app
// Define a function to calculate the sum
function calculateSum(a, b) {
return a + b;
}
// Add buttons to the page that trigger the function
const button1 = document.createElement('button');
button1.textContent = 'Add 2 numbers';
button1.addEventListener('click', () => {
const num1 = document.getElementById('num1Input').value;
const num2 = document.getElementById('num2Input').value;
const result = calculateSum(num1, num2);
document.getElementById('resultOutput').textContent = `The sum is ${result}`;
});
document.body.appendChild(button1);
const button2 = document.createElement('button');
button2.textContent = 'Add 3 numbers';
button2.addEventListener('click', () => {
const num1 = document.getElementById('num1Input').value;
const num2 = document.getElementById('num2Input').value;
const num3 = document.getElementById('num3Input').value;
const result = calculateSum(num1, num2, num3);
document.getElementById('resultOutput').textContent = `The sum is ${result}`;
});
document.body.appendChild(button2);
Tip 4: Join a Community
Learning JavaScript can be challenging at times, and having a community of like-minded individuals can be incredibly helpful. Look for online communities, such as Reddit’s r/javascript, Stack Overflow, or online forums where you can ask questions, share your projects, and get feedback from others.
Here’s an example of how you can join a community:
// Join a Reddit community
const reddit = new Reddit({
clientId: 'your-client-id',
clientSecret: 'your-client-secret',
username: 'your-username',
password: 'your-password'
});
reddit.login().then(() => {
const subreddit = reddit.subreddit('javascript');
subreddit.load().then(() => {
console.log('Logged in and loaded subreddit');
});
});
Tip 5: Use Online Resources
There are many online resources available that can help you learn JavaScript quickly and effectively. Some popular resources include Codecademy, Udemy, Coursera, and freeCodeCamp. These resources provide tutorials, videos, and exercises to help you learn at your own pace.
Here’s an example of how you can use online resources:
// Use Codecademy to learn JavaScript
const codecademy = new Codecademy({
clientId: 'your-client-id',
clientSecret: 'your-client-secret',
username: 'your-username',
password: 'your-password'
});
codecademy.login().then(() => {
const course = codecademy.course('javascript-fundamentals');
course.load().then(() => {
console.log('Logged in and loaded course');
});
});
Tip 6: Take Online Courses
Online courses are a great way to learn JavaScript quickly and effectively. Many online education platforms, such as Udemy, Coursera, and edX, offer courses on JavaScript and web development. These courses provide structured learning materials, video lectures, and exercises to help you learn at your own pace.
Here’s an example of how you can take an online course:
// Take a Udemy course on JavaScript
const udemy = new Udemy({
clientId: 'your-client-id',
clientSecret: 'your-client-secret',
username: 'your-username',
password: 'your-password'
});
udemy.login().then(() => {
const course = udemy.course('javascript-fundamentals');
course.load().then(() => {
console.log('Logged in and loaded course');
});
});
Tip 7: Use a JavaScript Editor
A JavaScript editor can help you write, debug, and optimize your code more efficiently. Some popular JavaScript editors include Sublime Text, Atom, and Visual Studio Code. These editors provide features such as syntax highlighting, code completion, and debugging tools that can help you save time and improve your productivity.
Here’s an example of how you can use a JavaScript editor:
// Use Sublime Text to write JavaScript code
const sublime = new SublimeText({
clientId: 'your-client-id',
clientSecret: 'your-client-secret',
username: 'your-username',
password: 'your-password'
});
sublime.login().then(() => {
const file = sublime.file('example.js');
file.open().then(() => {
console.log('Opened example.js in Sublime Text');
});
});
Tip 8: Learn by Doing Real-World Projects
One of the most effective ways to learn JavaScript is by doing real-world projects. Start by identifying a project that interests you, such as building a to-do list app or creating a simple game. Then, break down the project into smaller tasks and start working on them one by one. As you work on each task, you’ll learn new concepts and techniques that you can apply to future projects.
Here’s an example of how you can learn by doing real-world projects:
// Build a simple game using JavaScript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const game = {
// Define game variables and functions
score: 0,
objects: [],
draw: function() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
this.objects.forEach((object) => {
object.draw();
});
ctx.fillText(`Score: ${this.score}`, 10, 20);
},
update: function() {
this.objects.forEach((object) => {
object.update();
});
},
handleInput: function(event) {
switch (event.key) {
case 'ArrowUp':
this.score++;
break;
case 'ArrowDown':
this.score--;
break;
}
}
};
// Define game objects and functions
const ball = {
x: canvas.width / 2,
y: canvas.height / 2,
velocityX: 1,
velocityY: 1,
draw: function() {
ctx.beginPath();
ctx.arc(this.x, this.y, 10, 0, 2 * Math.PI);
ctx.fillStyle = 'red';
ctx.fill();
},
update: function() {
this.x += this.velocityX;
this.y += this.velocityY;
if (this.x > canvas.width - 10) {
this.velocityX = -1;
} else if (this.x < 10) {
this.velocityX = 1;
}
if (this.y > canvas.height - 10) {
this.velocityY = -1;
} else if (this.y < 10) {
this.velocityY = 1;
}
}
};
// Define game loop and event listeners
function updateGame() {
game.update();
requestAnimationFrame(updateGame);
}
document.addEventListener('keydown', (event) => {
game.handleInput(event);
});
requestAnimationFrame(updateGame);
Tip 9: Use Online Resources for Reference
As you learn JavaScript, it’s important to have access to online resources for reference. Some popular online resources include MDN Web Docs, W3Schools, and JavaScript Doc. These resources provide detailed documentation on JavaScript concepts, syntax, and APIs that you can use to enhance your knowledge.
Here’s an example of how you can use online resources for reference:
// Use MDN Web Docs to learn about the document object
const doc = document;
console.log(doc.documentElement); // Output: <html></html>
console.log(doc.body); // Output: <body></body>
Tip 10: Practice, Practice, Practice!
Finally, the key to mastering JavaScript is practice. Start by building small projects and gradually work your way up to more complex ones. As you practice, you’ll learn new concepts and techniques that you can apply to future projects.
Here’s an example of how you can practice JavaScript:
// Practice building a simple calculator app
const num1Input = document.getElementById('num1Input');
const num2Input = document.getElementById('num2Input');
const resultOutput = document.getElementById('resultOutput');
function calculateSum() {
const num1 = parseFloat(num1Input.value);
const num2 = parseFloat(num2Input.value);
const result = num1 + num2;
resultOutput.textContent = `The sum is ${result}`;
}
By following these tips and practicing regularly, you can quickly learn JavaScript and start building your own web applications.