How I Use JavaScript as a World-Class Developer
Unlock the power of JavaScript to enhance your web development skills. Learn how to use JavaScript to create interactive and dynamic web pages, with tips and tricks from a professional developer.
Updated: October 19, 2023
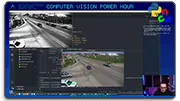
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!As a world-class JavaScript developer, I use JavaScript for a wide range of tasks, from building complex web applications to creating interactive client-side scripts. In this article, I’ll share some of my favorite techniques and tools for working with JavaScript, as well as provide code demonstrations to illustrate how I use them in real-world projects.
1. Modularizing Code with Namespaces
One of the most important principles of good software design is modularity, and this is especially true for JavaScript development. To keep my code organized and maintainable, I use namespaces to define my modules and separate concerns. Here’s an example of how I might create a namespace for a set of related functions:
// namespace.js
(function() {
'use strict';
// Define a module namespace
var myModule = {
// Define some functions and variables
greet: function(name) {
console.log('Hello, ' + name + '!');
},
sayGoodbye: function() {
console.log('Goodbye, world!');
}
};
// Export the namespace
module.exports = myModule;
})();
To use this namespace in another file, I can import it like so:
// main.js
(function() {
'use strict';
var myModule = require('./namespace');
// Use the functions and variables defined in the namespace
myModule.greet('Alice');
myModule.sayGoodbye();
})();
By using namespaces, I can keep my code organized and maintainable, even for large and complex projects.
2. Using Promises for Asynchronous Code
Asynchronous programming is a key aspect of JavaScript development, and one of the most popular tools for handling asynchronous code is the Promise
class. Here’s an example of how I might use Promises
to fetch some data from an API and display it in my UI:
// fetchData.js
(function() {
'use strict';
// Define a function that fetches data from an API
var fetchData = function(url) {
return new Promise(function(resolve, reject) {
// Make an HTTP request to the API
fetch(url)
.then(function(response) {
if (response.ok) {
// Resolve the promise with the response data
resolve(response.json());
} else {
// Reject the promise with an error message
reject('Failed to fetch data');
}
})
.catch(function(error) {
// Catch any errors that might occur during the fetch
console.log('Error fetching data:', error);
});
});
};
// Use the function to fetch some data from an API
var data = fetchData('https://api.example.com/data');
// Display the data in the UI
document.getElementById('data').innerHTML = JSON.stringify(data, null, 2);
})();
By using Promises
, I can handle asynchronous code in a much more straightforward and readable way than with callbacks or other approaches.
3. Utilizing Libraries and Frameworks
Finally, I want to touch on some of the libraries and frameworks that I use regularly as a world-class JavaScript developer. Some of my favorites include:
- React: A popular front-end library for building reusable UI components.
- Angular: A powerful front-end framework for building complex web applications.
- Vue.js: A flexible and easy-to-learn front-end framework that’s gaining popularity fast.
- Lodash: A utility library that provides a massive collection of functions for working with arrays, objects, and more.
- Moment.js: A popular library for working with dates and times in JavaScript.
By utilizing these libraries and frameworks, I can build applications much faster and with more reliability than if I were to write everything from scratch. Plus, I can leverage the expertise of a large community of developers who contribute to and maintain these tools.
I hope this article has given you some insight into how I use JavaScript as a world-class developer. Whether you’re just starting out or are an experienced dev yourself, I encourage you to experiment with these techniques and tools to take your own skills to the next level. Happy coding!