How to Learn JavaScript: A Guide for Beginners and Experts Alike
Learn JavaScript from scratch! Our comprehensive guide covers the basics of the language, including variables, data types, loops, and functions. Get started on your JavaScript journey today! (196 characters)
Updated: October 19, 2023
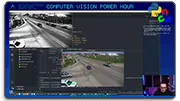
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Learning JavaScript can be a daunting task, especially for beginners. However, with the right resources and a bit of dedication, anyone can become proficient in this powerful programming language. In this article, we’ll cover some of the best ways to learn JavaScript, including code demonstrations and tips from experienced developers.
1. Start with the Basics
Before diving into advanced topics, it’s essential to understand the fundamentals of JavaScript. Here are a few key concepts to get you started:
a. Variables and Data Types
In JavaScript, variables are used to store values. There are several data types in JavaScript, including numbers, strings, booleans, objects, and arrays. Understanding these data types is crucial for building more complex applications.
let name = "John"; // string
let age = 25; // number
let isAdmin = true; // boolean
let users = [{"name": "John", "age": 25, "isAdmin": true}]; // object
b. Conditional Statements
Conditional statements are used to control the flow of your code based on certain conditions. Here are a few examples:
if (age > 18) {
console.log("You are eligible to vote");
} else {
console.log("You are not eligible to vote");
}
if (isAdmin === true) {
console.log("You have admin privileges");
} else {
console.log("You do not have admin privileges");
}
2. Get Familiar with JavaScript Syntax
JavaScript has a unique syntax that can take some getting used to. Here are a few examples of basic JavaScript syntax:
a. Functions
Functions are reusable blocks of code that perform a specific task. Here’s an example of a simple function:
function greet(name) {
console.log(`Hello, ${name}!`);
}
You can call this function by passing in a name as an argument:
greet("John"); // Output: Hello, John!
b. Loops and Conditional Statements
Loops and conditional statements are essential for building dynamic applications. Here’s an example of a for loop:
for (let i = 0; i < 5; i++) {
console.log(`Hello, world! ${i}`);
}
This code will output Hello, world! 0
, Hello, world! 1
, Hello, world! 2
, Hello, world! 3
, and Hello, world! 4
.
3. Learn Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that uses objects to represent data and functionality. JavaScript is an object-oriented language, so understanding OOP concepts is essential for building complex applications. Here are a few key concepts:
a. Classes and Objects
In JavaScript, classes and objects are used to define reusable blocks of code and data. Here’s an example of a simple class:
class Dog {
constructor(name) {
this.name = name;
}
bark() {
console.log("Woof!");
}
}
You can create an instance of this class by calling the Dog
constructor and passing in a name:
let fido = new Dog("Fido");
fido.bark(); // Output: Woof!
b. Inheritance
Inheritance is a OOP concept that allows you to create a new class based on an existing one. Here’s an example of inheritance in JavaScript:
class Dog extends Animal {
constructor(name) {
super(name);
}
bark() {
console.log("Woof!");
}
}
class Animal {
constructor(name) {
this.name = name;
}
makeSound() {
console.log("The animal makes a sound.");
}
}
In this example, the Dog
class extends the Animal
class, which means that all instances of Dog
will also be instances of Animal
. You can use the super
keyword to call the parent class’s methods.
4. Learn about Events and Listeners
Events and listeners are essential for building interactive applications in JavaScript. Here’s an example of how events work:
let button = document.getElementById("my-button");
button.addEventListener("click", function() {
console.log("Button clicked!");
});
In this example, we create a button element and add an event listener to it. When the button is clicked, the event listener function will be called, which logs “Button clicked!” to the console.
5. Practice, Practice, Practice!
The best way to learn JavaScript is by practicing. Start with simple projects and gradually work your way up to more complex applications. Here are a few project ideas:
a. Build a Simple Calculator
Create a simple calculator that takes in two numbers and performs basic arithmetic operations (e.g., addition, subtraction, multiplication, division).
b. Create a To-Do List App
Build a to-do list app that allows users to add, remove, and mark tasks as completed.
c. Build a Simple Game
Create a simple game (e.g., Tic-Tac-Toe, Snake) using JavaScript and HTML5 canvas.
6. Use Online Resources and Communities
There are many online resources and communities available to help you learn JavaScript. Here are a few recommendations:
a. Codecademy
Codecademy is an excellent resource for learning JavaScript, with interactive coding lessons and exercises.
b. FreeCodeCamp
FreeCodeCamp is a non-profit organization that offers a comprehensive curriculum in web development, including JavaScript.
c. YouTube
There are many YouTube channels dedicated to teaching JavaScript, such as Traversy Media and Scott Tolinski.
d. Reddit (r/javascript)
The r/javascript subreddit is an excellent community for asking questions and sharing knowledge with other JavaScript developers.
Conclusion
Learning JavaScript can be a challenging but rewarding experience. With the right resources and dedication, anyone can become proficient in this powerful programming language. Remember to start with the basics, practice regularly, and take advantage of online resources and communities to help you on your journey. Happy coding!