Mastering the Art of JavaScript: A Comprehensive Guide to Learning the Language and Taking Your Development Skills to the Next Level
Want to learn JavaScript? Our comprehensive guide covers everything you need to know, from basics to advanced techniques. Start your journey today and become a pro in no time!
Updated: October 19, 2023
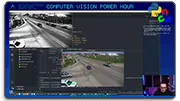
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript is a powerful programming language that is used by millions of developers around the world. It is a crucial skill for any web developer, and it can be used for a wide range of applications, from front-end web development to back-end server-side development. If you’re new to JavaScript, or if you want to improve your skills, this guide will help you get started.
Getting Started with JavaScript
Before you can start learning JavaScript, you need to have a basic understanding of HTML and CSS. These are the building blocks of the web, and they provide the structure and layout for your web pages. Once you have a solid foundation in these technologies, you can begin learning JavaScript.
Here are some resources to get you started:
- Codecademy’s JavaScript Course: This is an interactive course that covers the basics of JavaScript. It includes exercises and projects to help you practice your skills.
- MDN Web Docs: This is a comprehensive guide to JavaScript, provided by Mozilla. It covers everything from the basic syntax to advanced topics like async programming.
- W3Schools: This is another popular resource for learning JavaScript. It includes examples and reference materials for all of the major JavaScript concepts.
Learning the Basics of JavaScript
Once you have a solid foundation in HTML and CSS, it’s time to start learning the basics of JavaScript. Here are some key concepts to focus on:
- Variables and Data Types: In JavaScript, you can store data in variables, just like in any other programming language. There are several different data types that you can use, including numbers, strings, booleans, and objects.
// Example of a variable declaration
let myName = 'John Doe';
// Example of a data type
let age = 30;
- Conditional Statements: If statements and else statements are used to control the flow of your code based on certain conditions. For example, you might use an if statement to check if a user is logged in before displaying certain content.
// Example of an if statement
if (isLoggedIn) {
// Display content for logged-in users
} else {
// Display content for non-logged-in users
}
- Loops: Loops are used to repeat a block of code multiple times. There are two main types of loops in JavaScript: for loops and while loops.
// Example of a for loop
for (let i = 0; i < 5; i++) {
console.log(i);
}
// Example of a while loop
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
- Functions: Functions are blocks of code that perform a specific task. They can be called multiple times throughout your code, and they can take parameters to customize their behavior.
// Example of a function
function greet(name) {
console.log(`Hello, ${name}!`);
}
// Calling the function with a parameter
greet('John');
Advanced Topics in JavaScript
Once you have a solid foundation in the basics of JavaScript, it’s time to start exploring some of the more advanced concepts. Here are a few topics to focus on:
- Object-Oriented Programming (OOP): JavaScript is an object-oriented language, which means that it supports concepts like inheritance, polymorphism, and encapsulation. Understanding these concepts will help you write more robust and maintainable code.
// Example of a class
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
// Example of a constructor
let person1 = new Person('John', 30);
person1.sayHello(); // Output: Hello, my name is John and I am 30 years old.
// Example of inheritance
class Employee extends Person {
constructor(name, age, company) {
super(name, age);
this.company = company;
}
sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old, working for ${this.company}.`);
}
}
let employee1 = new Employee('John', 30, 'ABC Company');
employee1.sayHello(); // Output: Hello, my name is John and I am 30 years old, working for ABC Company.
- Async Programming: JavaScript is an asynchronous language, which means that it can handle multiple tasks at the same time. Understanding how to use async programming will help you write more efficient and responsive code.
// Example of async programming
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.log(error);
}
}
// Example of using async programming
let data = await fetchData();
console.log(data); // Output: The data returned from the API.
Conclusion
Learning JavaScript can be a challenging but rewarding experience. With these resources and this guide, you’ll be well on your way to becoming a proficient JavaScript developer. Remember to practice regularly, and don’t be afraid to try new things and experiment with different concepts. Happy coding!