Navigating the Treacherous Waters of JavaScript: Why It’s One of the Toughest Programming Languages to Master
Is learning JavaScript too hard? Get the inside scoop on the challenges of mastering this popular programming language and find out if it’s right for you.
Updated: October 19, 2023
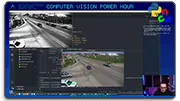
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Learning any programming language can be challenging, but some may argue that JavaScript is one of the most difficult languages to master. In this article, we’ll explore why JavaScript can be so tricky and provide some code demonstrations to help you understand the concepts better.
Syntax and Basics
Let’s start with the basics. JavaScript has a unique syntax that can be confusing for beginners. It’s a prototypal language, which means that objects can have properties and methods that are not defined explicitly. This can lead to unexpected behavior and errors if you’re not careful.
Here’s an example of a simple JavaScript program that outputs “Hello World!” to the console:
console.log("Hello World!");
This code is straightforward, but it already contains some of the key concepts that can be challenging for beginners:
console.log()
is a function that takes a string argument and prints it to the console.- The
;
character is used to separate statements, which means that each line of code must end with a semicolon. - There are no curly braces or explicit function definitions, which can make the code harder to read and understand.
Functions and Scope
Functions are an essential part of any programming language, and JavaScript is no exception. However, JavaScript’s function syntax can be confusing at first. Here’s an example of a simple function that takes two arguments and returns their sum:
function add(x, y) {
return x + y;
}
This code defines a function add
that takes two arguments x
and y
, and returns their sum. However, there are some potential pitfalls here:
- Functions in JavaScript can be defined using the
function
keyword, but they can also be defined using an arrow function expression. This can lead to confusion about which syntax to use when. - The
return
statement is used to return a value from a function, but it can only be used once per function. If you try to return multiple values, only the last one will be returned. - Functions in JavaScript have their own scope, which means that they can access variables and functions defined within their own scope, but not outside of it. This can lead to unexpected behavior if you’re not careful.
Object-Oriented Programming
JavaScript is an object-oriented language, which means that it supports encapsulation, inheritance, and polymorphism. However, these concepts can be challenging for beginners to grasp. Here’s an example of a simple class that represents a person:
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`);
}
}
This code defines a class Person
that takes two arguments name
and age
in its constructor. It also defines a sayHello
method that logs a message to the console. However, there are some potential pitfalls here:
- Classes in JavaScript can be defined using the
class
keyword, but they can also be defined using an object-oriented programming syntax. This can lead to confusion about which syntax to use when. - The
this
keyword is used to reference the current object instance, but it can only be used within the context of a function or method. If you try to use it outside of that context, you’ll get an error. - Inheritance and polymorphism are powerful features in JavaScript, but they can be challenging to understand and use correctly.
Conclusion
Learning JavaScript can be a challenging task, especially for beginners. The syntax and basics of the language can be confusing at first, and the object-oriented programming concepts can take time to grasp. However, with practice and dedication, anyone can learn to master JavaScript and build amazing web applications.
I hope this article has helped you understand some of the challenges of learning JavaScript and how to overcome them. Happy coding!