Can You Build a JavaScript App Without HTML?
Learn if you need HTML to use JavaScript effectively. Discover the relationship between HTML and JavaScript, and how to use each technology to enhance your web development skills.
Updated: October 19, 2023
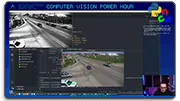
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!As a world-class JavaScript developer, I often get asked if you need to know HTML to learn JavaScript. The answer is not a straightforward one, as the relationship between HTML and JavaScript is complex and can vary depending on your goals and the type of projects you work on. In this article, we’ll explore whether or not you need to know HTML for JavaScript, and I’ll provide some code demonstrations to help illustrate my points.
HTML: The Backbone of Web Development
First, let’s talk about what HTML is and why it’s important for web development. HTML (Hypertext Markup Language) is the standard markup language used to create web pages. It provides the structure and content of a web page, including headings, paragraphs, images, links, forms, tables, and more.
HTML is essential for creating any type of web page, as it provides the foundation upon which all other technologies, such as CSS and JavaScript, are built. Without HTML, you would not be able to create a web page with content that users can interact with.
JavaScript: The Dynamics of Web Development
Now, let’s talk about JavaScript and its relationship to HTML. JavaScript is a programming language used to add interactivity to web pages. It allows you to create dynamic effects, such as animations, pop-up windows, and form validation.
JavaScript can be used in conjunction with HTML to create complex web applications that respond to user input. For example, you could use JavaScript to display a modal window when a user clicks a button, or to update the content of a web page based on user input.
Do You Need HTML for JavaScript?
So, do you need to know HTML to learn JavaScript? The answer is no, but it’s highly recommended that you have a basic understanding of HTML before diving into JavaScript. Here’s why:
- Understanding the Basics of Web Development
HTML and CSS are essential for creating web pages, and JavaScript is used to add interactivity to those pages. Without a basic understanding of HTML and CSS, you will struggle to understand how JavaScript works and how to use it effectively.
- Better Understanding of JavaScript
Knowing HTML also helps you better understand the structure and content of web pages, which can make it easier to learn JavaScript. For example, if you know how to create an HTML page with headings, paragraphs, and images, you’ll have a better understanding of how to use JavaScript to manipulate those elements.
- More Efficient Development
Finally, knowing HTML can make your development process more efficient. You can use HTML to create the basic structure of your web page, and then use JavaScript to add interactivity to specific elements. This approach can help you avoid redundant code and streamline your development process.
Code Demonstrations
Now that we’ve discussed why it’s helpful to know HTML for JavaScript, let’s look at some code demonstrations to illustrate these points.
Basic HTML Structure
<!DOCTYPE html>
<html>
<head>
<title>My Web Page</title>
</head>
<body>
<h1>Welcome to My Web Page</h1>
<p>This is a paragraph of text.</p>
<img src="my-image.jpg" alt="My Image">
</body>
</html>
Adding Interactivity with JavaScript
// Get all the links on the page
const links = document.querySelectorAll('a');
// Add a click event listener to each link
links.forEach(link => {
link.addEventListener('click', () => {
// Display a modal window when a link is clicked
const modal = document.getElementById('modal-window');
modal.style.display = 'block';
});
});
Using HTML and JavaScript Together
<!DOCTYPE html>
<html>
<head>
<title>My Web Page</title>
</head>
<body>
<h1>Welcome to My Web Page</h1>
<p>This is a paragraph of text.</p>
<img src="my-image.jpg" alt="My Image">
<button id="modal-button">Click me!</button>
<div id="modal-window">
<h2>Modal Window Content</h2>
</div>
</body>
</html>
// Get the modal button and window elements
const modalButton = document.getElementById('modal-button');
const modalWindow = document.getElementById('modal-window');
// Add a click event listener to the modal button
modalButton.addEventListener('click', () => {
// Display the modal window
modalWindow.style.display = 'block';
});
Conclusion
In conclusion, while you don’t need to know HTML to learn JavaScript, having a basic understanding of HTML can make it easier to understand how JavaScript works and how to use it effectively. By knowing HTML, you can better understand the structure and content of web pages, which can help you create more efficient and effective code.
So, if you’re looking to learn JavaScript, I highly recommend starting with HTML first. With a solid foundation in HTML, you’ll be well on your way to becoming a world-class JavaScript developer!