Do I Need JavaScript? Understanding the Role of JavaScript in Modern Web Development
Learn if you need JavaScript for your website, including its benefits and potential drawbacks. Discover how to determine if JavaScript is necessary for your site and how to use it effectively.
Updated: October 19, 2023
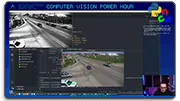
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript is a powerful programming language that can enhance the functionality of your website and provide a more interactive user experience. But do you really need JavaScript for your web application? In this article, we’ll explore some use cases where JavaScript is essential and others where it may not be necessary.
Essential Use Cases for JavaScript
- Interactive Elements: If your website has interactive elements like dropdown menus, tabs, accordions, or carousels, you need JavaScript to make them work smoothly. These elements rely on JavaScript’s ability to manipulate the Document Object Model (DOM) of the web page.
Here’s an example of a simple dropdown menu using JavaScript:
<button id="dropdown-btn">Dropdown</button>
<ul id="dropdown-list" class="hidden">
<li><a href="#">Option 1</a></li>
<li><a href="#">Option 2</a></li>
<li><a href="#">Option 3</a></li>
</ul>
// Get the dropdown button and list
const dropdownBtn = document.getElementById("dropdown-btn");
const dropdownList = document.getElementById("dropdown-list");
// Add an event listener to the button
dropdownBtn.addEventListener("click", function() {
// Toggle the visibility of the list
dropdownList.classList.toggle("hidden");
});
- Dynamic Content: If your website has dynamic content that changes based on user input or other factors, JavaScript is essential to make it work. For example, if you have a search bar that filters results based on user input, JavaScript is needed to handle the logic and update the page accordingly.
Here’s an example of a simple search function using JavaScript:
<form id="search-form">
<input type="text" id="search-input" placeholder="Search...">
<button id="search-btn">Search</button>
</form>
<div id="search-results"></div>
// Get the search form and input
const searchForm = document.getElementById("search-form");
const searchInput = document.getElementById("search-input");
// Add an event listener to the search button
const searchBtn = document.getElementById("search-btn");
searchBtn.addEventListener("click", function() {
// Get the user input from the search input field
const searchTerm = searchInput.value;
// Use JavaScript to fetch and display the search results
const searchResults = fetch(`https://example.com/search?q=${searchTerm}`)
.then(response => response.json())
.then(data => {
// Display the search results in the #search-results div
const resultsDiv = document.getElementById("search-results");
resultsDiv.innerHTML = "";
data.forEach(result => {
const resultElement = document.createElement("div");
resultElement.textContent = result.name;
resultsDiv.appendChild(resultElement);
});
});
});
- Single-Page Applications: If you’re building a single-page application (SPA) that loads content dynamically based on user input or other factors, JavaScript is essential to make it work. SPAs rely heavily on JavaScript to manipulate the DOM and handle user input.
Here’s an example of a simple SPA using JavaScript:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Single Page Application</title>
</head>
<body>
<h1 id="header">Welcome to our SPA!}</h1>
<p id="intro">This is a single page application built with JavaScript.</p>
<button id="load-btn">Load More Content</button>
<div id="content"></div>
<script src="app.js"></script>
</body>
</html>
// Get the header, intro, and content elements
const header = document.getElementById("header");
const intro = document.getElementById("intro");
const content = document.getElementById("content");
// Add an event listener to the load button
const loadBtn = document.getElementById("load-btn");
loadBtn.addEventListener("click", function() {
// Use JavaScript to fetch and display more content
const loadMoreBtn = document.getElementById("load-more-btn");
loadMoreBtn.innerHTML = "Loading...";
// Simulate a delay before loading the next set of content
setTimeout(() => {
const nextContent = `<p>This is some more content.</p>`;
content.innerHTML += nextContent;
loadMoreBtn.innerHTML = "Load More";
}, 2000);
});
Not essential Use Cases for JavaScript
- Static Web Pages: If your website is a simple static page with no interactivity or dynamic content, you may not need JavaScript at all. In this case, you can use HTML and CSS alone to create a functional website.
- Simple Forms: If your web application only has simple forms without any complex validation or logic, you may not need JavaScript. You can use HTML and CSS to style the form and handle user input with vanilla JavaScript.
- Basic Animations: If your web application only has basic animations that don’t rely on complex logic or dynamic content, you may not need JavaScript. You can use CSS alone to create simple animations.
Conclusion
In conclusion, JavaScript is an essential tool for web development, especially when it comes to creating interactive and dynamic web applications. However, not all websites require JavaScript, and some can function perfectly well with just HTML and CSS. The decision to use JavaScript should be based on the specific needs of your project and the complexity of your requirements.