Unit Testing with Jasmine
Learn the basics of unit testing in JavaScript with Jasmine. Explore the theory behind testing and discover multiple code examples. Improve your coding skills and ensure high-quality code with this beginner-friendly tutorial.
Updated: March 11, 2023
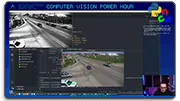
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Unit testing is a crucial part of software development, ensuring that each unit of code is functioning correctly in isolation before integrating it with other units of code. In the world of JavaScript, one of the most popular testing frameworks is Jasmine.
In this tutorial, we will introduce Jasmine and guide you through the process of setting up and running unit tests with Jasmine. By the end of this tutorial, you should have a basic understanding of how to write and run unit tests using Jasmine.
Prerequisites
To follow along with this tutorial, you should have a basic understanding of JavaScript, including variables, functions, and control flow statements. You should also have a code editor installed, such as Visual Studio Code or Sublime Text.
What is Jasmine?
Jasmine is an open-source testing framework for JavaScript that provides a simple and intuitive syntax for writing tests. It is a behavior-driven development (BDD) framework, which means that tests are written in a human-readable format that describes the behavior of the code being tested.
Jasmine can be used for both client-side and server-side JavaScript applications and is compatible with various JavaScript libraries and frameworks such as Angular, React, and Node.js.
Setting Up Jasmine
To use Jasmine, you need to download and include the Jasmine library in your project. You can download the library from the official Jasmine website or install it using a package manager such as npm or yarn.
For example, if you’re using npm, you can install Jasmine by running the following command:
npm install --save-dev jasmine
Once you have installed the Jasmine library, you need to create a file to contain your test suite. This file should be named with a .spec.js extension and be located in the same directory as the code you want to test.
Writing Tests with Jasmine
Tests in Jasmine are organized into suites and specs. A suite contains one or more specs, which are individual tests. You can use the describe function to create a suite and the it function to create a spec.
Here’s an example of a simple test suite and spec:
describe("Calculator", function() {
it("should add two numbers correctly", function() {
var result = add(2, 3);
expect(result).toEqual(5);
});
});
In this example, we have created a test suite for a calculator and a spec that tests the add function. The expect function is used to define the expected outcome of the test. In this case, we expect the result of adding 2 and 3 to equal 5.
Running Tests with Jasmine
To run the tests, you need to open the HTML file that includes the Jasmine library and your test suite file in a browser. Jasmine will automatically detect and run the tests.
You can also run the tests using a task runner or test runner, such as Grunt or Karma.
Conclusion
Unit testing is an essential part of software development that helps ensure the quality and reliability of your code. Jasmine is a popular and powerful testing framework for JavaScript that makes it easy to write and run tests. In this tutorial, we introduced Jasmine, showed you how to set it up, and walked you through writing and running tests with Jasmine.
Now that you have a basic understanding of Jasmine, you can start exploring more advanced testing techniques and practices to further improve the quality of your code. Happy testing!