Introduction to Vue
Learn the basics of Vue.js and how it works with our comprehensive tutorial. Explore the theory and code examples
Updated: March 11, 2023
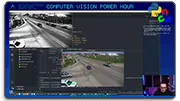
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Vue.js is a progressive JavaScript framework used for building user interfaces. It has become one of the most popular frameworks in the web development industry due to its simplicity and versatility. In this tutorial, we will cover the basics of Vue.js and how to use it to create interactive and dynamic web applications.
Getting Started with Vue.js
To get started with Vue.js, you need to include the Vue.js library in your HTML file. You can do this by including the following code in the head section of your HTML file:
<script src="https://cdn.jsdelivr.net/npm/vue"></script>
Once you have included the Vue.js library, you can create a new Vue instance by adding the following code in your JavaScript file:
javascript
Copy code
var app = new Vue({
el: '#app',
data: {
message: 'Hello Vue!'
}
})
In the code above, we create a new Vue instance and assign it to the variable app. The el property specifies the element in the HTML file that Vue will control. In this case, the element is the div with the id of “app”. The data property is an object that contains the data for our application. In this case, we have a property called message with the value “Hello Vue!”.
Using Directives in Vue.js
Directives are special attributes that allow you to bind Vue.js data to the HTML. They start with the “v-” prefix and are used to manipulate the DOM or to bind data to it. Let’s take a look at a few examples:
<div id="app">
<p v-if="showMessage">{{ message }}</p>
<input v-model="message">
</div>
In the code above, we have two directives. The v-if directive will only render the element if the showMessage property is true. The v-model directive binds the input field to the message property, so whenever the input value changes, the message property will be updated as well.
Handling User Input
Vue.js makes it easy to handle user input using the v-on directive. This directive is used to listen to DOM events and execute JavaScript code when they are triggered. Let’s take a look at an example:
<div id="app">
<button v-on:click="incrementCounter">Increment</button>
<p>{{ counter }}</p>
</div>
In the code above, we have a button that triggers the incrementCounter method when clicked. The counter property is then updated, and the updated value is displayed in the element.
var app = new Vue({
el: '#app',
data: {
counter: 0
},
methods: {
incrementCounter: function() {
this.counter++;
}
}
})
In the code above, we define the incrementCounter method that increments the counter property whenever it is called.
Conclusion
Vue.js is a powerful and intuitive JavaScript framework that allows you to create dynamic and interactive web applications. In this tutorial, we covered the basics of Vue.js, including creating a new Vue instance, using directives, and handling user input. With this knowledge, you should be able to start building your own applications using Vue.js.