Introduction to Express.js
Learn how to build web applications with Node.js using Express.js. This tutorial covers the basics of Express.js, including routing, middleware, and error handling, and includes code examples to help you get started
Updated: March 11, 2023
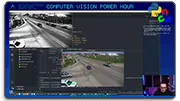
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Express.js is a popular web framework for Node.js that makes it easy to build web applications. It provides a simple, yet powerful, set of features that allow you to build applications quickly and easily. In this tutorial, we’ll cover the basics of Express.js, including routing, middleware, and error handling, and show you how to use them to build a simple web application.
First, let’s start with the basics. Express.js is a Node.js web framework that provides a set of features that allow you to build web applications quickly and easily. It is built on top of Node.js’s built-in HTTP module, which means it is fast and efficient.
It provides a number of features that make building web applications easier, including:
Routing: Express.js provides a simple and intuitive way to define routes for your web application. You can define routes based on HTTP methods (such as GET, POST, PUT, and DELETE), URL patterns, and query parameters.
Middleware: Express.js provides a middleware system that allows you to add functionality to your web application. Middleware functions are functions that can be executed before or after the request is processed, and can modify the request and response objects.
Error handling: Express.js provides a simple and powerful error handling mechanism that allows you to handle errors that occur in your application. You can define error handling middleware functions that will be executed when an error occurs.
Now, let’s look at some code examples. Here’s a simple example of how to create a web server using Express.js:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Server listening on port 3000');
});
In this example, we first require the Express.js module and create an instance of the express object. We then define a route for the root URL (/) using the app.get() method. This method takes two arguments: the URL pattern and a callback function that is executed when the route is matched.
The callback function takes two arguments: the request object (req) and the response object (res). In this example, we’re simply sending the string “Hello World!” back to the client using the res.send() method.
Finally, we start the server by calling the app.listen()
method and passing it the port number we want the server to listen on.
That’s a basic introduction to Express.js! In future tutorials, we’ll cover more advanced topics, such as template engines, authentication, and database integration. But for now, you should have a good understanding of the basics of Express.js and how to use it to build web applications with Node.js.