Introduction to React Building User Interfaces with JavaScript
Discover the fundamentals of React and how it works. This comprehensive guide explores the theory behind the library and includes multiple code examples, all written in a beginner-friendly style.
Updated: March 11, 2023
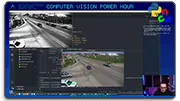
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!If you’re a JavaScript developer, you’ve probably heard of React - a popular library for building dynamic and interactive user interfaces. In this tutorial, we’ll explore what React is, how it works, and how to get started building your own web applications with it.
What is React?
React is a JavaScript library for building user interfaces. It was created by Facebook and is now maintained by a community of developers. React allows you to build complex UIs by breaking them down into smaller, reusable components.
How does React work?
React uses a virtual DOM (Document Object Model) to represent the UI. When a user interacts with your application, React updates the virtual DOM, which in turn updates the actual DOM. This process is known as reconciliation.
React components are JavaScript classes that contain render methods. Render methods describe what the UI should look like at any given moment in time, based on the current state and props of the component.
React also uses a unidirectional data flow, which means that data flows down from parent components to child components. If a child component needs to update data, it must do so through callbacks passed down from the parent component.
Getting started with React
To get started with React, you’ll need to install Node.js and npm (Node Package Manager) on your machine. Once you’ve done that, you can create a new React project using the create-react-app command:
npx create-react-app my-app
cd my-app
npm start
This will create a new React project in the my-app directory and start a development server.
Components and JSX
React components are at the core of building UIs with React. Components can be functional or class-based, and they can contain other components as well.
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript components. JSX is not required to use React, but it is a common way to build components.
Here’s an example of a simple React component that uses JSX:
import React from 'react';
function Welcome(props) {
return <h1>Hello, {props.name}!</h1>;
}
export default Welcome;
In this example, we define a functional component called Welcome that takes a prop called name and returns an h1 element with the name included.
State and Props
State and props are two concepts that are essential to understanding how React works.
State refers to the internal data of a component that can change over time. State is managed using the setState method, which updates the state of a component and triggers a re-render of the UI.
Props, on the other hand, are data that is passed down from parent components to child components. Props are immutable and cannot be changed by the child component.
Here’s an example of a React component that uses both state and props:
import React, { Component } from 'react';
class Counter extends Component {
state = {
count: 0
};
handleClick = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.handleClick}>Click me</button>
</div>
);
}
}
export default Counter;
In this example, we define a class-based component called Counter that contains a state property called count. We also define a handleClick method that updates the count when a button is clicked.
Conclusion
React is a powerful library for building dynamic and interactive user interfaces. In this tutorial, we’ve explored the basics of what React is, how it works, and how to get started building your own web applications with it.