An Introduction to Node.js: What It Is and How It Works
Learn the basics of Node.js and how it works. Discover how to create powerful server-side applications with JavaScript.
Updated: March 11, 2023
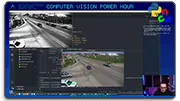
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Are you looking to learn about Node.js and how it can help you build scalable web applications? Look no further. In this article, we’ll cover the basics of Node.js, including what it is, how it works, and how to get started.
What is Node.js?
Node.js is an open-source, cross-platform, back-end JavaScript runtime environment that runs on the V8 engine. It allows developers to write server-side applications using JavaScript, a language traditionally used for front-end development.
How does Node.js work?
Node.js is built on top of the V8 JavaScript engine, which was originally developed for use in Google Chrome. It provides a powerful runtime environment for executing JavaScript code outside of a web browser.
One of the key features of Node.js is its event-driven, non-blocking I/O model. This means that Node.js can handle many concurrent connections without blocking the execution of other tasks. In other words, it can handle multiple requests at the same time, making it ideal for building real-time applications.
Node.js also comes with a built-in package manager called npm, which makes it easy to install and manage external libraries and modules.
Getting started with Node.js
To get started with Node.js, you’ll need to install it on your machine. Node.js is available for download on the official Node.js website.
Once you’ve installed Node.js, you can start creating and running Node.js applications using a text editor and the Node.js command line interface (CLI). To run a Node.js application, simply navigate to the directory containing the application in the terminal, and type “node app.js” (assuming your application is named app.js).
Here’s an example of a simple Node.js server that listens on port 3000:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!\n');
});
server.listen(3000, () => {
console.log('Server running on port 3000');
});
This code creates an HTTP server that listens on port 3000 and returns a “Hello, World!” message when a client makes a request.
Conclusion
Node.js is a powerful and flexible tool for building server-side applications using JavaScript. Its event-driven, non-blocking I/O model makes it ideal for real-time applications, and its built-in package manager (npm) makes it easy to manage external libraries and modules.
In this article, we’ve covered the basics of Node.js, including what it is, how it works, and how to get started. Now it’s up to you to explore Node.js further and see what you can build with it!