Introduction to Angular
Learn the basics of Angular and how it works in this comprehensive tutorial. Follow along with multiple code examples and start building dynamic web applications today.
Updated: March 11, 2023
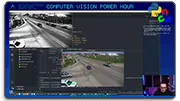
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!As web applications become increasingly complex, developers are seeking ways to create scalable, maintainable code that is easy to manage. One of the most popular frameworks for building web applications is Angular, a JavaScript-based platform that simplifies the development process by providing a variety of tools and features.
In this tutorial, we’ll explore the basics of Angular and how it works, as well as dive into some code examples. We’ll cover everything from setting up a new Angular project to creating components, services, and directives.
To get started, let’s first understand what Angular is and why it’s so popular.
What is Angular?
Angular is a JavaScript framework developed and maintained by Google. It was first released in 2010 and has since become one of the most widely used frameworks for building web applications. Angular allows developers to build dynamic, single-page applications that are scalable and easy to maintain.
One of the key features of Angular is its use of components. Components are the building blocks of an Angular application and are responsible for rendering the UI. Each component has its own template and styles, as well as a TypeScript class that defines its behavior.
Another important feature of Angular is its use of dependency injection. This allows developers to write modular, testable code by injecting dependencies into their components and services.
Now that we have an understanding of what Angular is and why it’s useful, let’s dive into some code examples. In the next section, we’ll cover how to set up a new Angular project.
Create your first Angular Project
To set up a new Angular project, we’ll need to use the Angular CLI. The CLI is a command-line interface that allows us to create and manage Angular projects. To install the CLI, open up a terminal and run the following command:
npm install -g @angular/cli
Once the CLI is installed, we can create a new project by running the following command:
ng new my-app
This will create a new Angular project called “my-app”. Once the project is created, we can run it by navigating into the project directory and running the following command:
cd my-app
ng serve --open
This will launch a development server and open up our new Angular application in the browser.
Next, let’s create a new component. Components are the building blocks of an Angular application and are responsible for rendering the UI. To create a new component, we’ll need to use the CLI again. Run the following command:
ng generate component my-component
This will create a new component called “my-component”. We can then use this component in our application by adding it to one of our existing components or by creating a new component that uses it.
Finally, let’s create a new service. Services are used to provide functionality that can be shared across multiple components. To create a new service, run the following command:
ng generate service my-service
This will create a new service called “my-service”. We can then inject this service into our components by adding it to the constructor function.
In this tutorial, we’ve covered the basics of Angular and how it works, as well as explored some code examples. While this is just the tip of the iceberg, we hope it has provided you with a solid foundation to start building your own Angular applications. Happy coding!