A Beginner’s Guide to Debugging in JavaScript
Learn how to debug JavaScript like a pro with our comprehensive guide. Understand the theory and master the tools and best practices for effective debugging.
Updated: March 11, 2023
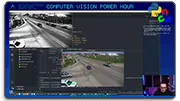
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!As a JavaScript developer, you will inevitably come across bugs in your code. Debugging is the process of finding and fixing these errors, and it’s an essential skill to have. In this article, we’ll explore the theory behind debugging in JavaScript and the tools you can use to make your life easier.
Part 1: Introduction to Debugging
Debugging is the process of identifying and fixing errors in code. It’s an essential skill for any developer, as it helps ensure that your code is working as intended. There are many different types of errors that can occur in JavaScript, including syntax errors, logical errors, and runtime errors.
Part 2: Debugging Techniques
When it comes to debugging JavaScript code, there are several techniques you can use to help identify and fix errors. These include console.log statements, breakpoints, and stepping through code. We’ll explore each of these in detail and provide code examples to help illustrate their use.
Here are some common techniques:
Using console.log() to print variables to the console:
function calculateSum(a, b) {
console.log("Calculating sum of " + a + " and " + b + "...");
let sum = a + b;
console.log("Sum is: " + sum);
return sum;
}
let x = 3;
let y = 7;
let result = calculateSum(x, y);
console.log("Result is: " + result);
Using breakpoints to pause code execution and inspect variables:
function calculateSum(a, b) {
let sum = a + b;
debugger; // <-- set a breakpoint here
return sum;
}
let x = 3;
let y = 7;
let result = calculateSum(x, y);
console.log("Result is: " + result);
Using the Chrome DevTools debugger to step through code:
function calculateSum(a, b) {
let sum = a + b;
return sum;
}
let x = 3;
let y = 7;
let result = calculateSum(x, y);
console.log("Result is: " + result);
In Chrome DevTools, you can set a breakpoint on the let result line, then use the Step Into, Step Over, and Step Out buttons to step through the calculateSum() function and inspect variables as you go.
Part 3: Debugging Tools
In addition to debugging techniques, there are also several tools you can use to make your life easier. These include the built-in debugger in your browser, as well as third-party tools like React Developer Tools and Redux DevTools. We’ll explore each of these tools and show you how to use them effectively.
Browser DevTools: Most modern web browsers come with built-in developer tools that allow developers to inspect and debug web applications. The DevTools provide a range of features, including console logging, breakpoints, network analysis, and DOM inspection.
Console: The console is a built-in tool in most web browsers that allows developers to log messages, view errors, and run commands. Developers can use console.log() statements to print debugging information to the console, and use other console methods to inspect variables and objects.
Debugger: JavaScript comes with a built-in debugger that allows developers to set breakpoints and step through code execution. The debugger can be accessed from the browser DevTools, or directly in a code editor like Visual Studio Code.
Linters: Linters are tools that analyze code for errors and potential problems. They can detect syntax errors, unused variables, and other issues that can lead to bugs. Popular linters for JavaScript include ESLint, JSHint, and JSLint.
Profilers: Profilers are tools that analyze code performance, identifying performance bottlenecks and areas for optimization. They can help developers identify slow or memory-intensive code, and suggest ways to optimize it. Popular profilers for JavaScript include Chrome DevTools' Performance tab and the Node.js profiler.
Third-party tools There are many third-party tools available for debugging JavaScript, including paid tools like Sentry and Raygun, and free tools like Bugsnag and Rollbar. These tools can help developers identify and fix errors in production code, and provide detailed error reports to help diagnose issues.
Part 4: Best Practices for Debugging
Finally, we’ll provide some best practices for debugging JavaScript code. These include writing modular and testable code, avoiding overly complex code, and using a consistent coding style. We’ll also discuss the importance of testing and how it can help prevent bugs from occurring in the first place.
debugging:
Use a consistent process: Develop a consistent and repeatable process for debugging, starting with reproducing the error, identifying the root cause, and then implementing a fix.
Check for syntax errors: Make sure to check for syntax errors using a linter or a tool like JSLint before debugging. Syntax errors can make it difficult to identify and solve other issues, so it’s important to catch them early.
Use console.log: Console.log is a powerful tool that allows developers to see what’s happening in their code at specific points in time. Using console.log can help identify errors and bugs and can also provide insight into how the code is functioning.
Use breakpoints: Breakpoints allow developers to pause the code at a specific point and step through it, line by line. This can be helpful for identifying exactly where an error is occurring and can also provide more information about the state of the code at that particular point.
Use debugging tools: There are many debugging tools available, including the built-in debugger in Chrome DevTools, Firebug, and Visual Studio Code. These tools offer features like step-by-step debugging, real-time code changes, and the ability to set watches on specific variables.
Document the debugging process: Keep track of the debugging process by documenting the steps taken to identify and fix the error. This can help in the future when similar errors occur, or when debugging with other developers.
Test thoroughly: After fixing an error, test the code thoroughly to ensure that the issue has been resolved and that no other issues have been introduced.
Conclusion:
Debugging is an essential skill for any JavaScript developer. By understanding the theory behind debugging, using the right techniques, and taking advantage of the available tools, you can save yourself time and frustration when trying to identify and fix errors in your code. Remember to follow best practices and keep testing in mind as you develop your applications.