Error Types and Handling
Learn how to handle errors in JavaScript with this comprehensive guide. Understand error types and explore various techniques for debugging and error handling in code.
Updated: March 11, 2023
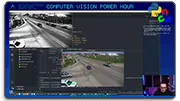
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!As a JavaScript developer, it’s essential to know how to handle errors in your code. Errors can occur at any time during the execution of your code, and if not handled properly, they can cause your program to crash or behave unexpectedly. In this article, we’ll explore the different types of errors in JavaScript and how to handle them effectively.
Types of Errors in JavaScript
JavaScript has several types of errors that can occur during the execution of your code. Here are some of the most common ones:
Syntax Errors: These occur when the code is not written correctly, and the interpreter cannot parse it.
Runtime Errors: These occur when a program tries to execute code that cannot be executed, such as calling a method on an undefined object or dividing by zero.
Logical Errors: These occur when the code runs without errors but produces incorrect results due to flawed logic or incorrect calculations.
Handling Errors in JavaScript
Now that we’ve covered the different types of errors, let’s look at how to handle them in JavaScript.
Try-Catch Blocks: One of the most common ways to handle errors in JavaScript is by using try-catch blocks. A try-catch block allows you to catch an error and handle it gracefully, without crashing your program.
For example:
try {
// some code
} catch (error) {
// handle error
}
Throwing Errors: You can also manually throw an error in your code using the throw keyword. This allows you to create custom error messages and handle them in a try-catch block.
For example:
function divideByZero(num) {
if (num === 0) {
throw new Error('Cannot divide by zero!');
}
return 10 / num;
}
try {
console.log(divideByZero(0));
} catch (error) {
console.error(error.message);
}
Error Objects: JavaScript provides built-in error objects that you can use to handle specific types of errors. Some of the most common ones are TypeError, ReferenceError, and RangeError.
Console Logging: Another useful technique for debugging errors in JavaScript is by using console logging. You can use console.log() or console.error() to output messages to the console, which can help you identify where the error occurred.
Conclusion
Errors are an inevitable part of writing JavaScript code, but by understanding the different types of errors and how to handle them, you can write more robust and reliable code. Whether you use try-catch blocks, error objects, or console logging, the key is to catch and handle errors gracefully, without crashing your program.
By following the techniques outlined in this article, you’ll be able to identify and fix errors in your JavaScript code quickly and efficiently, leading to more reliable and bug-free applications.