Debugging Techniques and Tools
Learn how to become a master debugger in JavaScript with this comprehensive guide. Discover the best techniques and tools for debugging your code and fix errors with ease.
Updated: March 11, 2023
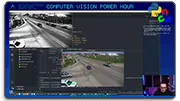
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Debugging is an essential part of the software development process, and JavaScript is no exception. Even the most experienced developers encounter bugs in their code, and fixing them can be a challenging task. However, with the right tools and techniques, debugging can become a lot easier.
In this article, we’ll explore the theory behind debugging in JavaScript, the different types of bugs you may encounter, and the tools and techniques available for debugging your code.
The Theory Behind Debugging
In general, debugging is the process of identifying and fixing errors in your code. The goal of debugging is to identify the root cause of the problem and then make the necessary changes to fix it.
In JavaScript, the most common types of bugs are syntax errors, runtime errors, and logical errors.
Syntax errors happen when you have a mistake in your code that violates the syntax rules of the language. For example, if you forget to close a parenthesis or use an incorrect keyword, the JavaScript interpreter will report a syntax error.
Runtime errors happen when your code attempts to execute an operation that’s not allowed or when it tries to access an undefined value. These types of errors can be harder to spot, as they often don’t cause the code to fail immediately.
Logical errors are bugs that don’t cause your code to fail, but they produce unexpected or incorrect results. These types of errors can be the most challenging to identify, as they may not be apparent from the code alone.
Debugging Tools and Techniques
JavaScript provides several built-in tools for debugging, such as console.log, debugger statements, and error messages. Here’s a brief overview of each:
console.log: This method allows you to print values to the console, which can be useful for debugging. You can use it to check the value of a variable or to see if a particular block of code is executing.
debugger statements: This is another useful tool for debugging. You can insert a debugger statement in your code to pause the execution at that point and allow you to inspect the code.
error messages: JavaScript provides error messages when it encounters syntax or runtime errors. These messages can provide valuable information about what went wrong and where the error occurred.
In addition to these built-in tools, there are several third-party debugging tools available. For example, the Chrome DevTools provide a comprehensive suite of debugging tools that allow you to inspect and debug your JavaScript code.
Best Practices for Debugging
Here are some best practices to keep in mind when debugging your JavaScript code:
Keep your code simple: The simpler your code is, the easier it will be to debug. Try to break your code down into small, manageable chunks, and test each piece independently.
Use console.log: This method is your best friend when it comes to debugging. Use it to print out values and check if your code is executing as expected.
Test your code in different environments: Different browsers can interpret JavaScript differently, so it’s essential to test your code in different environments to ensure it works as expected.
Use meaningful variable names: Using descriptive variable names can make it easier to understand what’s happening in your code and can help you track down bugs more quickly.
Conclusion
Debugging is an essential part of the software development process, and JavaScript is no exception. By understanding the theory behind debugging, the different types of bugs you may encounter, and the tools and techniques available, you’ll be well-equipped to identify and fix bugs in your code. Remember to keep your code simple, use console.log, test in different environments, and use meaningful variable names to make the debugging process as smooth as possible.