What is the DOM?
Learn how the Document Object Model (DOM) works in JavaScript and how it’s used to manipulate HTML and CSS. Explore the theory and multiple code examples to understand the DOM deeply.
Updated: March 11, 2023
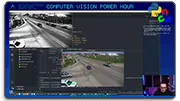
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!The Document Object Model (DOM) is a crucial concept in web development. It’s a programming interface for HTML and XML documents, which represent web pages in browsers. In simple terms, it’s a way for JavaScript to interact with HTML and CSS.
The DOM is a hierarchical structure of objects that represent all the elements in an HTML document. When a web page is loaded, the browser creates a Document Object Model of the page. The DOM consists of various objects that represent the document, such as the document object, which represents the entire page.
Each HTML element in the document is represented by an object in the DOM. For example, a paragraph is represented by a “p” object, a link is represented by an “a” object, and so on. These objects can be accessed and manipulated using JavaScript.
One of the most significant benefits of the DOM is that it allows developers to manipulate HTML and CSS using JavaScript. This means that developers can dynamically modify the content and appearance of a web page based on user interaction or other events.
To access and manipulate the DOM, developers use various methods and properties provided by JavaScript. For example, the “getElementById()” method is used to select an element by its ID attribute. The “innerHTML” property is used to access or modify the content of an element. The “style” property is used to access or modify the CSS properties of an element.
Let’s look at an example of how to modify the DOM using JavaScript. Suppose we have an HTML page with a button and a paragraph. We want to change the content of the paragraph when the button is clicked. Here’s the code:
HTML:
<button id="myBtn">Click me</button>
<p id="myPara">Hello, world!</p>
JavaScript:
const myBtn = document.getElementById("myBtn");
const myPara = document.getElementById("myPara");
myBtn.addEventListener("click", () => {
myPara.innerHTML = "You clicked the button!";
});
In the above code, we first select the button and paragraph elements using the “getElementById()” method. We then add an event listener to the button that listens for a “click” event. When the button is clicked, the content of the paragraph is changed to “You clicked the button!” using the “innerHTML” property.
Conclusion
The DOM is an essential concept in web development. It allows developers to interact with and modify HTML and CSS using JavaScript. Understanding how the DOM works is crucial for creating dynamic and responsive web pages.