Selecting DOM Elements
Learn how to select DOM elements with JavaScript and take your web development skills to the next level. This comprehensive guide includes theory and multiple code examples to help you master the topic.
Updated: March 11, 2023
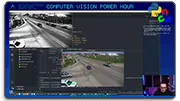
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript is a versatile language that allows web developers to create dynamic and interactive web pages. One of the most fundamental concepts of web development with JavaScript is DOM manipulation, which involves modifying and selecting DOM elements. In this article, we’ll explore how to select DOM elements with JavaScript in detail.
Before diving into the specifics of selecting DOM elements, let’s first understand what the Document Object Model (DOM) is. The DOM is a programming interface for web documents that represents the page so that programs can change the document structure, style, and content. Essentially, the DOM provides a structured representation of the HTML document, which can be modified and updated using JavaScript.
To select DOM elements with JavaScript, you’ll need to use one of the many built-in methods available. The most common method is the querySelector method, which returns the first element that matches a specified CSS selector. Here’s an example:
const element = document.querySelector('.my-class');
In this example, we’re using the querySelector method to select an element with the class name my-class. This method returns the first element that matches the specified selector.
Another method that you can use to select DOM elements is the getElementById method, which returns the element with the specified ID. Here’s an example:
const element = document.getElementById('my-id');
In this example, we’re using the getElementById method to select an element with the ID my-id.
There are several other methods available for selecting DOM elements with JavaScript, including getElementsByClassName, getElementsByName, and getElementsByTagName. Each method has its own unique syntax and use cases, so it’s important to familiarize yourself with all of them.
Once you’ve selected a DOM element with JavaScript, you can then modify it using various methods and properties. For example, you can change the text content of an element using the textContent property, like this:
const element = document.querySelector('.my-class');
element.textContent = 'New Text Content';
In this example, we’re selecting an element with the class name my-class and then updating its text content to “New Text Content”.
You can also modify the attributes of an element using the setAttribute method, like this:
const element = document.querySelector('.my-class');
element.setAttribute('src', 'new-image.jpg');
In this example, we’re selecting an element with the class name my-class and then updating its src attribute to point to a new image file.
In conclusion, selecting DOM elements with JavaScript is an essential skill for any web developer. By mastering the various methods and properties available, you can create dynamic and interactive web pages that engage your users. Keep practicing and experimenting with different techniques, and you’ll soon become a master of DOM manipulation with JavaScript!