Modifying DOM Elements
Learn how to modify DOM elements in JavaScript with ease! This comprehensive guide includes theory, practical examples, and beginner-friendly explanations.
Updated: March 11, 2023
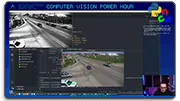
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!The Document Object Model (DOM) is a programming interface that allows us to access and manipulate HTML and XML documents. Modifying DOM elements with JavaScript is a powerful technique that enables us to create dynamic and interactive web applications. In this article, we will dive into the theory of how modifying DOM elements with JavaScript works, and provide multiple code examples to help you understand the concepts deeply.
How Modifying DOM elements in JavaScript Works
The DOM is organized as a hierarchical tree structure, where each element is represented by a node. These nodes can be accessed and manipulated using JavaScript. When a web page loads, the browser creates a DOM tree by parsing the HTML code. Once the DOM tree is created, we can use JavaScript to access, add, modify, or remove any element on the page.
Modifying DOM elements with JavaScript involves several steps:
Select the element you want to modify:
We can select an element by using its ID, class name, tag name, or any other attribute. This is typically done using the document.querySelector() or document.getElementById() methods.
Modify the element:
Once we have selected the element, we can modify its attributes, style, text content, or HTML content using JavaScript. For example, we can change the background color of a div element, add a new paragraph to a section, or update the text of a button.
Apply the changes:
After modifying the element, we need to apply the changes to the DOM tree. This is typically done using the appendChild(), replaceChild(), or insertBefore() methods.
Code Examples
Let’s look at some code examples to better understand how to modify DOM elements with JavaScript.
Example 1: Change the background color of a div element.
<div id="myDiv">Hello, World!</div>
const myDiv = document.getElementById('myDiv');
myDiv.style.backgroundColor = 'red';
Explanation:
In this example, we first select the div element with the ID “myDiv” using the document.getElementById() method. Then we use the style property to change the background color to red.
Example 2: Add a new paragraph to a section.
<section id="mySection">
<h2>Section Title</h2>
<p>Paragraph 1</p>
</section>
const mySection = document.getElementById('mySection');
const newParagraph = document.createElement('p');
newParagraph.textContent = 'Paragraph 2';
mySection.appendChild(newParagraph);
Explanation:
In this example, we first select the section element with the ID “mySection”. Then we create a new paragraph element using the document.createElement() method and set its text content to “Paragraph 2”. Finally, we use the appendChild() method to add the new paragraph to the section.
Example 3: Update the text of a button.
<button id="myButton">Click Me</button>
const myButton = document.getElementById('myButton');
myButton.textContent = 'Click Me Now';
Explanation:
In this example, we first select the button element with the ID “myButton”. Then we use the textContent property to update the text of the button to “Click Me Now”.
Conclusion:
Modifying DOM elements with JavaScript is a fundamental skill that every web developer should master. By understanding how to manipulate the DOM tree, we can create dynamic and interactive web pages that respond to user input and change over time. In this article, we discussed the theory of how modifying DOM elements with JavaScript works and provided multiple code examples to help you practice and apply these concepts in your own projects. Keep learning and practicing, and you will become a DOM manipulation pro in no time