Event Handling in the DOM
Learn how to handle DOM events efficiently with JavaScript. This comprehensive guide covers theory and multiple code examples. Suitable for beginners.
Updated: March 11, 2023
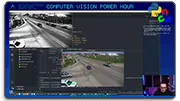
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!As JavaScript is becoming more and more popular for building web applications, it’s important to understand how to handle events in the Document Object Model (DOM). This article will explore the theory behind DOM event handling and provide examples of how to handle different types of events in JavaScript.
Understanding the Document Object Model (DOM):
The Document Object Model (DOM) is a tree-like structure that represents the HTML document as a hierarchy of objects. The DOM is an API for web developers that allows them to manipulate the structure, style, and content of web pages using JavaScript.
Event Handling in the DOM:
An event is any action that occurs on a web page, such as a button click or a key press. Event handling is the process of responding to these events in JavaScript.
Event listeners are used to register functions that will be called when a specific event occurs. Event listeners are attached to a specific element in the DOM and can be used to respond to events such as clicks, key presses, or form submissions.
Example:
// add an event listener to a button
const button = document.querySelector('#myButton');
button.addEventListener('click', () => {
console.log('Button clicked');
});
The addEventListener()
method is used to attach an event listener to the button element. The first argument is the name of the event to listen for (in this case, click), and the second argument is the function to be called when the event occurs.
Event Propagation:
When an event occurs on an element in the DOM, it is also triggered on all of its parent elements. This is known as event propagation.
Event propagation can be divided into two phases: the capturing phase and the bubbling phase. During the capturing phase, the event is first captured by the outermost element and then propagated down to the target element. During the bubbling phase, the event is propagated up from the target element to the outermost element.
Example:
// add event listeners to a div and a button inside the div
const div = document.querySelector('#myDiv');
const button = document.querySelector('#myButton');
div.addEventListener('click', () => {
console.log('Div clicked');
}, true); // capture phase
button.addEventListener('click', () => {
console.log('Button clicked');
});
In this example, we add a click event listener to both the div and button elements. The div element has a third argument, true, which specifies that the event should be captured during the capturing phase.
Conclusion:
DOM event handling is a fundamental part of building dynamic web applications with JavaScript. Understanding how events work in the DOM and how to handle them efficiently can make your code more robust and user-friendly. By using event listeners and understanding event propagation, you can create more responsive and interactive web applications.