Using Strict Mode in JavaScript
Learn about using strict mode in JavaScript and how it can help you write cleaner, safer code. Explore the theory and practical examples in this informative tutorial
Updated: March 11, 2023
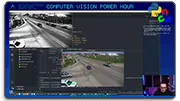
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript has come a long way since its inception, and the introduction of strict mode is one of the key enhancements that have been made to the language. Strict mode is a special mode that enables you to write more secure and efficient code in JavaScript. In this chapter, we’ll explore the benefits of using strict mode in your code, how it works, and multiple code examples that demonstrate its effectiveness.
What is Strict Mode in JavaScript?
Strict mode is a way to opt into a restricted variant of JavaScript that enforces stricter rules and more secure coding practices. In other words, it’s a way to ensure that your code is written with more attention to detail and with fewer errors.
How Does Strict Mode Work?
When you enable strict mode, you’re telling the JavaScript engine to execute your code in a stricter way. This means that certain JavaScript features that were previously allowed will now be disallowed or produce errors. For example, in strict mode, you must declare all variables using the var, let, or const keywords, otherwise, a ReferenceError will be thrown. Similarly, you can’t use the with statement or delete variables that are not explicitly declared.
The Benefits of Using Strict Mode
Using strict mode comes with many benefits. It makes your code more secure by preventing certain common programming errors, such as accidentally declaring global variables or using undefined variables. It also makes your code more efficient by disabling certain features that slow down the JavaScript engine. Additionally, strict mode makes it easier to optimize your code for performance, as it prevents certain types of optimizations from being made.
Examples of Strict Mode in Action
Let’s look at some examples of strict mode in action.
Preventing Global Variables
In non-strict mode, it’s possible to accidentally create global variables by forgetting to declare them with the var keyword. In strict mode, this is disallowed, as the following code demonstrates:
function myFunction() {
'use strict';
x = 10; // ReferenceError: x is not defined
}
Disallowing Duplicate Parameters
In non-strict mode, it’s possible to define multiple function parameters with the same name. In strict mode, this is disallowed, as the following code demonstrates:
function myFunction(x, y, x) {
'use strict';
// SyntaxError: Duplicate parameter name not allowed in this context
}
Preventing Octal Syntax
In non-strict mode, it’s possible to use the octal syntax by prefixing a number with a zero. In strict mode, this is disallowed, as the following code demonstrates:
function myFunction() {
'use strict';
var x = 010; // SyntaxError: Octal literals are not allowed in strict mode.
}
Enabling Strict Mode
To enable strict mode, you can add the ‘use strict’ directive at the beginning of your script or function. For example:
'use strict';
function myFunction() {
// Code in strict mode
}
You can also enable strict mode for an individual function by adding the directive at the beginning of the function.
Conclusion
In conclusion, strict mode is an incredibly powerful tool that can help you write more secure, efficient, and optimized code in JavaScript. By enabling strict mode, you can prevent common programming errors, write more efficient code, and optimize your code for performance. I highly recommend using strict mode in your JavaScript projects to take advantage of these benefits.