Using Efficient Data Structures in JavaScript
Learn how to use efficient data structures in JavaScript to optimize your code. This comprehensive guide covers best practices for using arrays, objects, and maps, along with built-in methods. Suitable for beginners.
Updated: March 11, 2023
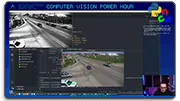
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Efficient data structures are a crucial aspect of writing high-performance JavaScript code. In this chapter, we’ll explore how efficient data structures work in JavaScript, and how you can use them to improve the speed and efficiency of your code.
What are Data Structures in JavaScript?
Data structures are a way of organizing and storing data in a computer’s memory. In JavaScript, there are several built-in data structures that can be used to store and retrieve data, including arrays, objects, and maps.
Why are Efficient Data Structures Important?
Efficient data structures are important for several reasons:
- They can improve the performance and speed of your JavaScript code.
- They can reduce the amount of memory and CPU usage required by your code.
- They can make it easier to organize and manipulate complex data sets.
- Best Practices for Using Efficient Data Structures
Here are some best practices for using efficient data structures in your JavaScript code:
Use arrays for sequential data - Arrays are an efficient way to store and manipulate sequential data, such as lists of items.
Use objects for key-value pairs - Objects are an efficient way to store and retrieve data using key-value pairs.
Use maps for complex data - Maps are an efficient way to store and manipulate complex data sets that require unique keys and values.
Avoid using nested data structures - Nested data structures can be inefficient and difficult to work with.
Use built-in methods for manipulating data - JavaScript provides several built-in methods for manipulating data structures, such as push(), pop(), and splice().
Example of Efficient Data Structures
Here’s an example of how to use an efficient data structure to improve the performance of your code:
// Non-optimized code
let myArray = [1, 2, 3, 4, 5];
for (let i = 0; i < myArray.length; i++) {
console.log(myArray[i]);
}
// Optimized code
let myArray = [1, 2, 3, 4, 5];
myArray.forEach(function(item) {
console.log(item);
});
In this example, we’ve optimized the loop by using the forEach() method of the array, which is a built-in method that allows you to iterate over each element of the array. This can be faster and more efficient than using a traditional for loop.
Summary
Using efficient data structures is an important aspect of writing high-performance JavaScript code. By following best practices and using the appropriate data structures for your needs, you can improve the efficiency and performance of your code, making it faster and more responsive for your users.