JavaScript Efficient Algorithms
write a meta tag description for the article that doesn’t have marketing speak, but uses active voice and is under 255 characters
Updated: March 11, 2023
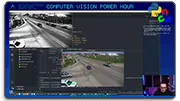
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Efficient algorithms are crucial for developing high-performance JavaScript applications. In this chapter, we’ll explore some popular algorithms and data structures that can help you optimize your code.
How Do Efficient Algorithms Work?
Efficient algorithms are designed to perform tasks with the minimum amount of resources, such as time and memory. These algorithms achieve their efficiency through techniques such as recursion, dynamic programming, and divide-and-conquer.
By using efficient algorithms and data structures, you can minimize the number of computations required to perform a task, reduce memory usage, and speed up your code.
Popular Algorithms and Data Structures
Here are some popular algorithms and data structures that can help you optimize your JavaScript code:
Sorting algorithms - Sorting algorithms like QuickSort and MergeSort can help you sort arrays of data quickly and efficiently.
Search algorithms - Search algorithms like Binary Search can help you find items in sorted arrays more efficiently than linear search.
Hash tables - Hash tables provide a way to store and access data with constant time complexity, making them ideal for applications that require frequent lookups.
Trees - Trees are hierarchical data structures that can be used for a variety of purposes, such as organizing data or implementing search algorithms.
Graphs - Graphs are collections of nodes and edges that can be used to model complex relationships between objects.
Example of Using Efficient Algorithms with JavaScript
Here’s an example of how to use a sorting algorithm in JavaScript:
// Using QuickSort to sort an array of numbers
function quickSort(arr) {
if (arr.length <= 1) return arr;
const pivot = arr[arr.length - 1];
const left = [];
const right = [];
for (let i = 0; i < arr.length - 1; i++) {
if (arr[i] < pivot) {
left.push(arr[i]);
} else {
right.push(arr[i]);
}
}
return [...quickSort(left), pivot, ...quickSort(right)];
}
const unsortedArray = [10, 5, 2, 3, 7, 6, 8, 9, 1, 4];
const sortedArray = quickSort(unsortedArray);
console.log(sortedArray); // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
In this example, we’ve used the QuickSort algorithm to sort an array of numbers. By using an efficient sorting algorithm like QuickSort, we can sort large arrays quickly and with minimal resources.
Efficient Algorithms in JavaScript
Bubble Sort: A simple sorting algorithm that compares adjacent elements and swaps them if they are in the wrong order. This algorithm has a time complexity of O(n^2) in the worst case, but is still commonly used for small data sets.
Merge Sort: A divide-and-conquer sorting algorithm that divides the input array into smaller sub-arrays, sorts them recursively, and then merges them back together. This algorithm has a time complexity of O(n log n) in the worst case, making it much more efficient than bubble sort for larger data sets.
Binary Search: An algorithm for searching through a sorted array by repeatedly dividing the search interval in half. This algorithm has a time complexity of O(log n) in the worst case, making it very efficient for large arrays.
Kadane’s Algorithm: An algorithm for finding the maximum sum of a subarray within an array of numbers. This algorithm has a time complexity of O(n) in the worst case, making it a very efficient way to solve this problem.
Sieve of Eratosthenes: An algorithm for finding all prime numbers up to a given limit. This algorithm has a time complexity of O(n log log n) in the worst case, making it one of the most efficient algorithms for finding prime numbers.
These are just a few examples of efficient algorithms in JavaScript. There are many more out there, and understanding and implementing them can greatly improve the performance of your code.
Conclusion
By using efficient algorithms and data structures, you can optimize your JavaScript code and improve its performance.