JavaScript Security Best Practices
Learn how to protect your JavaScript code from common web attacks like XSS and CSRF. Follow best practices for validation, sanitization, HTTPS, and API security. Suitable for beginners.
Updated: March 11, 2023
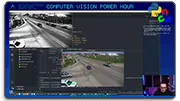
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Security is a critical concern in web development, and JavaScript is no exception. In this chapter, we’ll explore some best practices for keeping your JavaScript code secure and protecting your users.
How Does JavaScript Security Work?
JavaScript security involves protecting your web application from various attacks, such as cross-site scripting (XSS), cross-site request forgery (CSRF), and injection attacks. These attacks can result in the theft of user data, the compromise of your web application, or the spread of malicious content.
To prevent these attacks, you need to follow some best practices for secure coding, such as validating user input, sanitizing data, and using secure APIs.
Best Practices for JavaScript Security
Here are some best practices for keeping your JavaScript code secure:
Validate user input - Ensure that user input is validated on both the client and server sides to prevent attacks such as SQL injection.
Sanitize data - Remove potentially harmful code or characters from user input to prevent XSS attacks.
Use HTTPS - Ensure that your web application uses HTTPS to encrypt data in transit and prevent man-in-the-middle attacks.
Use secure APIs - Use secure APIs that require authentication and limit access to sensitive data.
Keep your code up-to-date - Stay up-to-date with the latest security patches and vulnerabilities and update your code accordingly.
Example of JavaScript Security Best Practices
Here’s an example of how to use JavaScript security best practices to prevent XSS attacks:
// Without sanitization
const userInput = '<script>alert("XSS attack!");</script>';
const output = document.getElementById('output');
output.innerHTML = userInput;
// With sanitization
const userInput = '<script>alert("XSS attack!");</script>';
const output = document.getElementById('output');
output.textContent = userInput;
In this example, we’ve sanitized the user input by using the textContent property instead of the innerHTML property to prevent the execution of potentially harmful code.
By following best practices for JavaScript security, you can ensure that your web applications are secure and protect your users' data from malicious attacks.