Minimize DOM Manipulation in JavaScript
Learn about minimizing DOM manipulation in JavaScript to improve your web application performance. Best practices include using templates, batch DOM operations, and event delegation. Suitable for beginners.
Updated: March 11, 2023
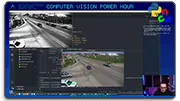
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Minimizing DOM manipulation in JavaScript is an important technique that can improve the performance of your web applications. In this chapter, we’ll explore the theory behind how DOM manipulation affects performance and some best practices for minimizing it.
How Does DOM Manipulation Affect Performance?
DOM manipulation refers to the process of changing the structure or content of a web page using JavaScript. While DOM manipulation is a powerful feature, it can also have a significant impact on the performance of your web applications.
When you manipulate the DOM, the browser needs to recalculate the layout and repaint the affected parts of the page, which can be a time-consuming process. In addition, frequent DOM manipulation can cause your application to become unresponsive or slow.
Best Practices for Minimizing DOM Manipulation
Here are some best practices for minimizing DOM manipulation in your JavaScript code:
Use templates - Use a template engine like Handlebars or Mustache to generate HTML from data, rather than creating HTML elements in JavaScript.
Batch DOM operations - Instead of making frequent DOM updates, batch them together into a single operation to reduce the number of layout and repaint cycles.
Use CSS classes - Instead of manipulating individual DOM elements, use CSS classes to apply styles to groups of elements.
Use event delegation - Instead of attaching an event listener to every element, use event delegation to listen for events on a parent element and handle them on the appropriate child elements.
Example of Minimizing DOM Manipulation in JavaScript
Here’s an example of how to use templates to minimize DOM manipulation in your JavaScript code:
// Without templates
const data = {
name: 'John',
age: 30
};
const container = document.getElementById('container');
const nameElement = document.createElement('h1');
const ageElement = document.createElement('p');
nameElement.textContent = data.name;
ageElement.textContent = data.age;
container.appendChild(nameElement);
container.appendChild(ageElement);
// With templates
const data = {
name: 'John',
age: 30
};
const template = Handlebars.compile(`
<h1>{{name}}</h1>
<p>{{age}}</p>
`);
const container = document.getElementById('container');
container.innerHTML = template(data);
In this example, we’ve used a template engine (Handlebars) to generate HTML from data, rather than creating HTML elements in JavaScript. This reduces the amount of DOM manipulation needed, improving the performance of our web application.
By following best practices for minimizing DOM manipulation in your JavaScript code, you can create web applications that are faster and more responsive, providing a better user experience.