JavaScript Security Best Practices
Learn about JavaScript optimizers and how they can improve your code performance. Explore popular tools like UglifyJS, Closure Compiler, Babel, and V8. Suitable for beginners.
Updated: March 11, 2023
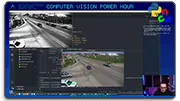
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!JavaScript optimizers are tools that can improve the performance and efficiency of your JavaScript code. In this chapter, we’ll explore the theory behind how optimizers work and some popular examples of JavaScript optimizers.
How Do JavaScript Optimizers Work?
JavaScript optimizers work by analyzing your code and making changes to improve its performance. They use several techniques, including:
- Minification: Removing unnecessary characters from your code, such as white space and comments, to reduce its size and improve loading time.
- Tree shaking: Removing unused code from your application, to reduce its size and improve loading time.
- Inlining: Replacing function calls with the actual code they contain, to reduce overhead and improve performance.
- Just-in-time (JIT) compilation: Compiling your code at runtime, to improve performance and reduce load times.
Popular JavaScript Optimizers
Here are some popular JavaScript optimizers:
UglifyJS - A JavaScript minifier that removes unnecessary characters from your code.
Closure Compiler - A JavaScript compiler that uses tree shaking and other techniques to optimize your code.
Babel - A JavaScript transpiler that converts modern JavaScript code into a version that is compatible with older browsers.
V8 - A JavaScript engine that includes a JIT compiler, to improve the performance of JavaScript applications.
Example of Using JavaScript Optimizers
Here’s an example of how to use UglifyJS to optimize your JavaScript code:
// Original code
function addNumbers(a, b) {
return a + b;
}
// Optimized code
function addNumbers(a,b){return a+b;}
In this example, we’ve used UglifyJS to optimize our JavaScript code by removing unnecessary characters from our function. This can reduce the size of our code and improve loading time.
Conclusion
JavaScript optimizers are powerful tools that can help you improve the performance and efficiency of your JavaScript code. By using techniques such as minification, tree shaking, inlining, and JIT compilation, you can create JavaScript applications that are faster and more efficient.