JavaScript Code Organization
Improve your JavaScript code with best practices for code organization. Learn about modular programming, code splitting, naming conventions, and more. Suitable for beginners.
Updated: March 11, 2023
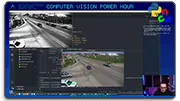
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Code organization is an essential aspect of writing maintainable and efficient JavaScript code. In this chapter, we’ll explore some best practices for organizing your code, including modular programming, code splitting, and naming conventions.
Why is Code Organization Important?
Code organization is important for several reasons:
- It makes your code more readable and easier to maintain, by breaking it down into smaller, more manageable modules.
- It reduces the risk of naming collisions and conflicts with other parts of your code or external libraries.
- It can improve the performance and loading time of your application by using code splitting and lazy loading.
Best Practices for Code Organization
Here are some best practices for organizing your JavaScript code:
Use modular programming - Break your code down into small, reusable modules, and organize them into separate files or folders.
Use code splitting - Split your code into smaller chunks and load them only when needed, to improve performance and reduce loading time.
Use consistent naming conventions - Use clear, consistent naming conventions for variables, functions, and modules, to make your code easier to read and understand.
Use comments and documentation - Use comments and documentation to explain how your code works, and make it easier for others to understand and maintain.
Example of Code Organization
Here’s an example of how to organize your JavaScript code using modular programming:
// Non-optimized code
function calculateArea(radius) {
return Math.PI * radius * radius;
}
function calculateCircumference(radius) {
return 2 * Math.PI * radius;
}
// Optimized code
const circle = {
calculateArea(radius) {
return Math.PI * radius * radius;
},
calculateCircumference(radius) {
return 2 * Math.PI * radius;
}
};
export default circle;
In this example, we’ve optimized the code by using modular programming, which separates the code into smaller, reusable modules. We’ve also used clear naming conventions and comments to make the code more readable and easier to understand.
Conclusion
Code organization is an essential aspect of writing maintainable and efficient JavaScript code. By following best practices such as modular programming, code splitting, and naming conventions, you can create code that is easier to maintain, more efficient, and more readable.