Avoid Global Variables
Learn best practices for avoiding global variables in JavaScript. This chapter covers function scope, module patterns, local variables, and strict mode. Improve code quality and efficiency with these tips. Suitable for beginners.
Updated: March 11, 2023
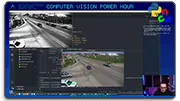
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Avoiding global variables is an important aspect of writing high-quality JavaScript code. In this chapter, we’ll explore why global variables can be problematic, and some best practices for avoiding them in your code.
What are Global Variables in JavaScript?
Global variables are variables that are defined outside of a function or block scope, and can be accessed from anywhere in your code. While global variables can be convenient to use, they can also be problematic for several reasons:
- They can create naming collisions, where two variables with the same name are used in different parts of your code.
- They can be accessed and modified by any part of your code, making it difficult to track changes and debug problems.
- They can lead to inefficiencies in your code, as they can take up memory and processing power even when they’re not being used.
Why is Avoiding Global Variables Important?
Avoiding global variables is important for several reasons:
- It can reduce the risk of naming collisions and conflicts with other parts of your code or external libraries.
- It can improve the modularity and maintainability of your code, by making it easier to isolate and test individual components.
- It can make your code more efficient, by reducing the amount of memory and processing power required to run your program.
Best Practices for Avoiding Global Variables
Here are some best practices for avoiding global variables in your JavaScript code:
Use function scope - Declare variables inside functions, rather than in the global scope.
Use module patterns - Use module patterns to create self-contained modules that don’t pollute the global namespace.
Use local variables - Use local variables instead of global variables to store data and share information between functions.
Use strict mode - Use the strict mode in JavaScript to enforce better coding practices and catch errors.
Example of Avoiding Global Variables
Here’s an example of how to avoid using global variables in your code:
// Non-optimized code
let myVar = 5;
function myFunction() {
console.log(myVar);
}
myFunction();
// Optimized code
function myFunction() {
let myVar = 5;
console.log(myVar);
}
myFunction();
In this example, we’ve optimized the code by declaring the variable inside the function, rather than in the global scope. This reduces the risk of naming collisions and makes it easier to isolate and test the function.
Conclusion
Avoiding global variables is an important aspect of writing high-quality JavaScript code. By following best practices and using function scope and module patterns, you can create more efficient, modular, and maintainable code that is easier to work with and debug.