Variables and Datatypes
Master variables and data types in JavaScript with this comprehensive chapter. Learn how to define variables, work with different data types, and understand type coercion and variable scope. Suitable for beginners.
Updated: March 11, 2023
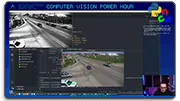
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Variables and data types are essential components of JavaScript programming. Variables are used to store values, and data types define the type of data that can be stored in a variable. In this chapter, we’ll explore how variables and data types work in JavaScript, and how they can be used to build complex programs.
Defining Variables
In JavaScript, you can define a variable using the keyword “let”. Here’s an example:
let x = 10;
In this example, the variable x is defined and assigned the value 10.
Data Types
There are several data types in JavaScript, including strings, numbers, booleans, arrays, objects, and more. Here are some examples:
let name = "John"; // string
let age = 30; // number
let isStudent = true; // boolean
let hobbies = ["reading", "writing", "coding"]; // array
let person = {firstName:"John", lastName:"Doe"}; // object
In this example, variables are defined for different data types, including a string, a number, a boolean, an array, and an object.
Type Coercion
JavaScript has a feature called type coercion, which allows the conversion of one data type to another. Here’s an example:
let x = "10";
let y = 5;
console.log(x + y); // Outputs "105"
In this example, the variable x is a string, but the + operator is used to perform concatenation with the number 5. JavaScript automatically converts the number 5 to a string and concatenates it with the string “10”.
Variable Scope
In JavaScript, variables can have either global or local scope. Global variables are defined outside of functions and can be accessed from anywhere in the program, while local variables are defined inside of functions and can only be accessed within that function. Here’s an example:
let x = 10; // Global variable
function myFunction() {
let y = 5; // Local variable
console.log(x + y); // Outputs 15
}
myFunction();
In this example, the global variable x is defined outside of the function, and the local variable y is defined inside the function. The console.log() statement inside the function accesses both variables to output 15.
Conclusion
Variables and data types are the building blocks of JavaScript programming. By understanding how to define variables, work with different data types, and use type coercion and variable scope, you can take your programming skills to the next level and write more powerful and efficient code.