Mastering Multidimensional Arrays in JavaScript: A Beginner’s Guide
Explore the power of multidimensional arrays in JavaScript with this comprehensive tutorial. Learn how to create, access, and manipulate complex data structures. Take your programming skills to the next level with this beginner-friendly guide.
Updated: March 14, 2023
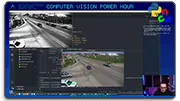
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Hello, fellow JavaScript coders! Today, we’re going to dive into the fascinating world of multidimensional arrays in JavaScript. As always, I’ll do my best to keep things simple and engaging so that even beginners can grasp these essential concepts. So, buckle up and let’s get started!
What are Multidimensional Arrays?
In JavaScript, an array is a data structure that can hold a collection of values, usually called elements. These elements can be of any data type, like numbers, strings, objects, or even other arrays. When an array contains other arrays as its elements, we call it a multidimensional array.
Think of a multidimensional array as a matrix or a table with rows and columns. For instance, a two-dimensional array represents a table with rows and columns, and a three-dimensional array can be visualized as a cube.
Creating a Multidimensional Array
In JavaScript, there’s no built-in support for multidimensional arrays. However, we can create them using nested arrays. Let’s see how we can create a 2D array:
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
Here, we’ve created a 3x3 matrix using an array of arrays. The outer array contains three arrays, each representing a row, and these inner arrays have three elements each, representing the columns.
Now, if we want to access an element from the matrix, we can use the following syntax:
console.log(matrix[1][2]); // Output: 6
In this example, matrix1 returns the second row (remember, arrays are zero-indexed), and 2 after that accesses the third element in that row, which is 6.
Working with Multidimensional Arrays
Let’s now explore some common operations we might want to perform on multidimensional arrays.
Iterating Over Elements To loop through all the elements of a 2D array, we can use nested for loops like this:
let rows = matrix.length;
let cols = matrix[0].length;
for (let i = 0; i < rows; i++) {
for (let j = 0; j < cols; j++) {
console.log(matrix[i][j]);
}
}
This code will output all the elements in the matrix one by one.
Adding Elements
To add elements to a multidimensional array, we can use the push method like we would with a regular array. Let’s add a new row to our matrix:
matrix.push([10, 11, 12]);
Now, our matrix looks like this:
[
[1, 2, 3],
[4, 5, 6],
[7, 8, 9],
[10, 11, 12]
]
Removing Elements
We can remove elements from a multidimensional array using the splice method. To remove the last row from the matrix, we can do the following:
matrix.splice(matrix.length - 1, 1);
Now, our matrix is back to its original form:
[
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
And there you have it!
Frequently asked questions
What is a multidimensional array in JavaScript?
A multidimensional array is an array containing one or more arrays within it. These nested arrays can have their own arrays, allowing for multiple levels of data storage, resembling a matrix or a table.
How do you define a multidimensional array?
In JavaScript, a multidimensional array is defined just like a regular array; the difference lies in the elements it contains. The elements are arrays themselves. Example:
let multiArray = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
Can you have arrays with different lengths in a multidimensional array?
Yes, JavaScript arrays are dynamic, and each sub-array in a multidimensional array can have a different length. This is known as a “jagged” array.
How do you access elements in a multidimensional array?
Elements in a multidimensional array can be accessed using multiple indices. The first index refers to the initial array, and the subsequent indices refer to the elements within the inner arrays. Example: multiArray0 would access the element 2 in the array provided in A2.
How do you loop through a multidimensional array?
You can loop through a multidimensional array by using nested loops. An outer loop iterates through the main array while an inner loop goes through each individual sub-array.
Can you use methods like push or pop on multidimensional arrays?
Yes, methods like push and pop can be used with multidimensional arrays, but they will affect only the array you are directly interacting with, either the main array or one of the sub-arrays, depending on the syntax.
Is there a limit to the number of dimensions in JavaScript arrays?
JavaScript does not explicitly limit the number of dimensions, but practical constraints like memory and processing power can limit the number of dimensions you can effectively manage and navigate.
How do you add items to a multidimensional array?
To add items to a specific array within a multidimensional array, target it with indices and use methods like push for adding elements. Example: multiArray1.push(10); would add the number 10 to the second array in multiArray.
Can multidimensional arrays contain different types of data?
Yes, similar to regular arrays, multidimensional arrays can store different types of data, including strings, numbers, objects, and even functions, within different sub-arrays.
How can I remove an element from a sub-array in a multidimensional array?
You can remove elements from a sub-array using methods like splice(), targeting the sub-array with the appropriate indices. Example: multiArray[2].splice(1, 1);
would remove the second element from the third array in multiArray.
Understanding multidimensional arrays is essential for handling complex data structures in programming.
Conclusion
And there you have it! We’ve learned the basics of multidimensional arrays in JavaScript. In conclusion, multidimensional arrays are a crucial tool for any JavaScript programmer. With the knowledge gained from this tutorial, you can now confidently work with multidimensional arrays in your JavaScript projects and take your programming skills to the next level.