Loops in JavaScript
write a meta tag description for the article that doesn’t have marketing speak, but uses active voice and is under 255 characters
Updated: March 11, 2023
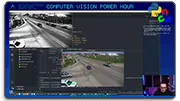
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Loops are a fundamental part of JavaScript programming. They allow you to execute a block of code multiple times, based on certain conditions or criteria. In this chapter, we’ll dive deep into how loops work in JavaScript and explore some examples of how they can be used.
For Loops
For loops are used to execute a block of code a specific number of times. They are commonly used to iterate over arrays or perform a specific task a certain number of times. Here’s an example:
for (let i = 0; i < 5; i++) {
console.log(i);
}
In this example, the for loop will iterate 5 times, starting with i = 0 and incrementing i by 1 each time, until i is greater than or equal to 5. The code inside the for loop console.log(i)
will be executed each time the loop runs, printing the value of i to the console.
While Loops
While loops are used to execute a block of code as long as a certain condition is true. They are commonly used when you don’t know how many times a loop needs to run. Here’s an example:
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
In this example, the while loop will continue to run as long as i is less than 5. The code inside the while loop (console.log(i) and i++) will be executed each time the loop runs, printing the value of i to the console and incrementing i by 1.
Do-While Loops
Do-while loops are similar to while loops, but they guarantee that the code inside the loop will be executed at least once, even if the condition is false. Here’s an example:
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
In this example, the do-while loop will run once, printing the value of i to the console (which is 0), and then check the condition. Since i is less than 5, the loop will continue to run, printing the values of i (1, 2, 3, and 4) to the console.
Loop Control Statements
JavaScript also provides several loop control statements that allow you to control the behavior of loops. These include the break statement, which immediately exits the loop, and the continue statement, which skips the current iteration of the loop and moves on to the next one. Here’s an example of using the break statement:
for (let i = 0; i < 10; i++) {
if (i === 5) {
break;
}
console.log(i);
}
In this example, the for loop will iterate 10 times, printing the values of i to the console. However, when i reaches 5, the break statement is executed, causing the loop to immediately exit.
Conclusion
Loops are a powerful feature of JavaScript programming that can greatly enhance the flexibility and efficiency of your code. By understanding how to use for loops, while loops, do-while loops, and loop control statements, you can take your programming skills to the next level and write more powerful and efficient code.