Arrays in JavaScript
Learn how to work with arrays in JavaScript with this comprehensive tutorial. Explore the creation, modification, and iteration of arrays, and discover the power of multidimensional arrays. Take your programming skills to the next level with this beginner-friendly guide.
Updated: March 11, 2023
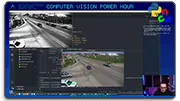
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Welcome to the world of JavaScript programming! In this tutorial, we’re going to explore arrays in JavaScript in depth.
Arrays are a powerful feature of JavaScript that allow you to store multiple values in a single variable. They are essential for organizing and manipulating data in your programs.
Creating Arrays
To create an array in JavaScript, use square brackets [] and separate each value with a comma. For example:
var fruits = ["Apple", "Banana", "Orange", "Mango"];
This code creates an array called “fruits” that contains four strings: “Apple”, “Banana”, “Orange”, and “Mango”.
Accessing Array Values
You can access the values in an array using their index number. In JavaScript, arrays are zero-indexed, which means that the first value in an array is at index 0, the second value is at index 1, and so on. For example:
var firstFruit = fruits[0];
console.log(firstFruit);
This code sets the variable “firstFruit” to the value at index 0 in the “fruits” array (which is “Apple”), and then logs that value to the console.
Modifying Array Values
You can modify the values in an array by assigning a new value to a specific index. For example:
fruits[1] = "Grapes";
console.log(fruits);
This code changes the value at index 1 in the “fruits” array from “Banana” to “Grapes”, and then logs the updated array to the console.
Adding and Removing Array Values
You can add or remove values from an array using various methods. For example, the “push” method adds a value to the end of an array:
fruits.push("Pineapple");
console.log(fruits);
This code adds the value “Pineapple” to the end of the “fruits” array and logs the updated array to the console.
The “pop” method removes the last value from an array:
fruits.pop();
console.log(fruits);
This code removes the last value from the “fruits” array (which is “Pineapple”) and logs the updated array to the console.
Iterating Over Arrays
You can iterate over the values in an array using a loop. For example, the following code logs each value in the “fruits” array to the console:
for (var i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
This code uses a “for” loop to iterate over each index in the “fruits” array. It then logs the value at each index to the console.
Multidimensional Arrays
JavaScript also supports multidimensional arrays, which are arrays that contain other arrays. For example:
var matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
This code creates a multidimensional array called “matrix” that contains three arrays, each with three values.
To access a value in a multidimensional array, you need to use two index numbers. For example, the following code logs the value at index [1][2] in the “matrix” array to the console:
console.log(matrix[1][2]);
This code uses two index numbers to access the value at index 2 in the second array (which is 6).
Conclusion
Arrays are a fundamental and versatile data structure in JavaScript programming. By understanding how to create, access, modify, and iterate over arrays, you can effectively store and manipulate data in your programs.
JavaScript also supports multidimensional arrays that allow you to store complex data structures with ease. With the knowledge and skills gained from this tutorial, you can now confidently work with arrays in your JavaScript projects and take your programming skills to the next level.