Prototypes and Inheritance
Discover the power of prototypes and inheritance in JavaScript with our in-depth guide. Learn how they work and gain a solid understanding with real-world examples. Perfect for beginners and advanced programmers alike.
Updated: March 11, 2023
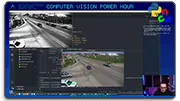
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!If you’re a JavaScript developer, you’ve probably heard of prototypes and inheritance. They’re fundamental concepts that can make your code more efficient and organized. In this article, we’ll explore what they are and how they work in detail.
Prototypes:
In JavaScript, every object has a prototype. A prototype is a blueprint for creating other objects. When you create an object, it inherits properties and methods from its prototype. The prototype chain is a series of linked prototypes that are used to create new objects. Let’s see how this works with an example:
// Create a prototype object
let animal = {
sound: "roar"
};
// Create a new object that inherits from the animal prototype
let lion = Object.create(animal);
console.log(lion.sound); // Output: "roar"
In this example, we created a prototype object called animal that has a property called sound. We then created a new object called lion that inherits from the animal prototype using the Object.create() method. When we access the sound property of the lion object, it looks up the prototype chain and finds the sound property in the animal prototype.
Inheritance:
Inheritance is the process of creating a new object that inherits properties and methods from an existing object. In JavaScript, inheritance is achieved through prototypes. Let’s see an example of inheritance in action:
// Create a prototype object
let vehicle = {
drive: function() {
console.log("Driving...");
}
};
// Create a car object that inherits from the vehicle prototype
let car = Object.create(vehicle);
// Add a new method to the car object
car.stop = function() {
console.log("Stopping...");
};
car.drive(); // Output: "Driving..."
car.stop(); // Output: "Stopping..."
In this example, we created a prototype object called vehicle that has a method called drive(). We then created a new object called car that inherits from the vehicle prototype using the Object.create() method. We added a new method called stop() to the car object. When we call the drive() and stop() methods on the car object, it looks up the prototype chain and finds the drive() method in the vehicle prototype and the stop() method in the car object.
Conclusion:
Prototypes and inheritance are important concepts in JavaScript. They help you create more efficient and organized code. Understanding how they work can help you write better JavaScript code. We hope this article has helped you gain a beginner-friendly understanding of prototypes and inheritance in JavaScript.