JavaScript Object Oriented Programming
Discover the power of object-oriented programming with Javascript. Learn the fundamentals and explore practical examples.
Updated: March 11, 2023
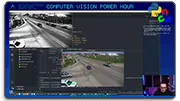
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Object-oriented programming (OOP) is a programming paradigm that emphasizes the use of objects and classes to organize and structure code. In JavaScript, OOP allows you to create objects that have properties and methods, making it a powerful tool for building complex applications.
The Basics of OOP in JavaScript
In JavaScript, everything is an object. This includes primitive data types such as strings and numbers, as well as arrays and functions. Objects in JavaScript are created using constructor functions, which define the properties and methods of the object.
Here is an example of a constructor function in JavaScript:
function Person(name, age) {
this.name = name;
this.age = age;
this.greet = function() {
console.log("Hello, my name is " + this.name);
}
}
This function creates a Person object that has two properties (name and age) and a method (greet). To create a new Person object, you can use the new keyword:
var person1 = new Person("John", 30);
var person2 = new Person("Jane", 25);
In this example, person1 and person2 are both instances of the Person object. They each have their own name and age properties, as well as the greet method.
Inheritance in OOP
One of the core principles of OOP is inheritance. Inheritance allows you to create a new object that is a modified version of an existing object. In JavaScript, you can achieve inheritance using prototype chains.
Here is an example of inheritance in JavaScript:
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + " makes a noise.");
}
function Dog(name) {
Animal.call(this, name);
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.speak = function() {
console.log(this.name + " barks.");
}
var d = new Dog("Rufus");
d.speak(); // outputs "Rufus barks."
In this example, we define an Animal object that has a speak method. We then create a new Dog object that inherits from Animal. The Dog object overrides the speak method to bark instead of making a generic noise.
Encapsulation in OOP
Another important concept in OOP is encapsulation. Encapsulation is the practice of hiding the implementation details of an object from the outside world, and only exposing a public interface.
In JavaScript, you can achieve encapsulation using closure functions. Here is an example:
function Counter() {
var count = 0;
this.increment = function() {
count++;
}
this.getCount = function() {
return count;
}
}
var counter = new Counter();
counter.increment();
console.log(counter.getCount()); // outputs 1
In this example, we define a Counter object that has a private count variable. The increment and getCount methods are defined inside the constructor function using closure, so they have access to the private count variable. This allows us to encapsulate the implementation details of the Counter object and only expose a public interface.
Conclusion
Object-oriented programming is a powerful tool for building complex applications in JavaScript. By using constructor functions, inheritance, and encapsulation, you can create objects that are modular, reusable, and easy to maintain