Hoisting in JavaScript
Understand hoisting in JavaScript with our in-depth guide. Learn how hoisting works and avoid common mistakes in your code.
Updated: March 11, 2023
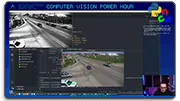
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!Have you ever been confused by the behavior of variables in JavaScript? Maybe you tried to access a variable before it was declared, and it still worked. This phenomenon is known as hoisting, and it can be a source of confusion for many JavaScript developers.
In this article, we’ll explore what hoisting is, how it works, and why it matters in JavaScript. We’ll cover the theory behind hoisting, dive into examples of how it works in practice, and discuss some best practices for avoiding common pitfalls.
What is Hoisting?
So, what exactly is hoisting? At a high level, hoisting is the behavior in JavaScript where variable and function declarations are moved to the top of their respective scopes during compilation, regardless of where they are actually written in the code.
For example, consider the following code:
console.log(myVar); // undefined
var myVar = "Hello, world!";
Even though myVar is not declared before it is used, this code will not throw an error. Instead, undefined will be logged to the console. This is because the var declaration is hoisted to the top of its scope during compilation.
However, it’s important to note that only the declaration is hoisted, not the initialization. For example:
console.log(myVar); // undefined
var myVar;
myVar = "Hello, world!";
This code produces the same output as the previous example, even though the declaration and initialization are split into two separate lines.
Hoisting can also occur with function declarations, as shown in this example:
sayHello(); // "Hello, world!"
function sayHello() {
console.log("Hello, world!");
}
Even though sayHello()
is called before it is declared, this code still works because the function declaration is hoisted to the top of its scope.
However, hoisting does not work the same way with function expressions, which are not hoisted. For example:
sayHello(); // TypeError: sayHello is not a function
var sayHello = function() {
console.log("Hello, world!");
}
In this code, sayHello is initialized with a function expression, which is not hoisted. As a result, trying to call sayHello() before it is initialized will result in a TypeError.
Understanding hoisting is important for writing clean and maintainable JavaScript code. Here are some best practices to keep in mind:
- Always declare your variables and functions before using them to avoid unexpected behavior.
- Avoid using function expressions before they are defined, as they are not hoisted and can lead to errors.
- Use let and const instead of var to avoid some of the quirks associated with hoisting in JavaScript.
Conclusion
In conclusion, hoisting is a powerful feature in JavaScript that can help simplify your code, but it’s important to understand how it works in order to avoid unexpected behavior.
By following best practices and writing clean code, you can avoid some of the common pitfalls associated with hoisting and write better JavaScript.