Closures and Scope
Learn the ins and outs of closures and scope in JavaScript. From the basics to advanced techniques, this guide will help you understand how they work and how to use them to write more efficient and maintainable code
Updated: March 11, 2023
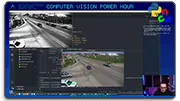
This YouTube channel is packed with awesome videos for coders!
Tons of videos teaching JavaScript and coding. Cool live streams!
Click Here to check out the Channel!As you dive deeper into JavaScript, you’ll come across the concepts of closures and scope. While they may seem confusing at first, they are essential to understanding how JavaScript works and writing efficient code.
What are Closures?
So what exactly are closures and scope in JavaScript? Simply put, scope refers to where a variable is available in your code, while a closure is a function that has access to variables from its outer (enclosing) function.
What is Scope?
Understanding scope is critical to avoiding conflicts with variable names and making sure that your code is organized and efficient. In JavaScript, there are two types of scope: global scope and local scope.
Global Scope
Global scope refers to variables that are available throughout your entire codebase. These variables are accessible from anywhere in your code, including from within functions.
Local Scope
Local scope, on the other hand, refers to variables that are only accessible within a specific function or block of code. These variables are created when a function is executed and are destroyed when the function exits.
Now that you understand scope, let’s dive into closures. Closures are functions that have access to variables from their outer function, even after that function has completed execution.
Closures
In JavaScript, closures are created whenever a function is declared inside another function. This allows the inner function to access variables from the outer function, even after the outer function has completed execution.
Closures are powerful because they allow you to create private variables and functions that are only accessible from within a specific function. This can help you write more efficient and maintainable code, as it reduces the risk of variable conflicts and allows you to organize your code more effectively.
Here’s an example of a closure in action:
function outerFunction() {
const outerVariable = "I'm from outer function";
function innerFunction() {
console.log(outerVariable);
}
return innerFunction;
}
const innerFunc = outerFunction();
innerFunc(); // logs "I'm from outer function"
In this example, the inner function has access to the outerVariable from the outer function, even after the outer function has completed execution.
Conclusion
Understanding closures and scope in JavaScript is critical to writing efficient and maintainable code. By using closures to create private variables and functions and understanding the differences between global and local scope, you’ll be able to take your JavaScript skills to the next level.